In a django app, how do I prevent users from deleting content not created by them?
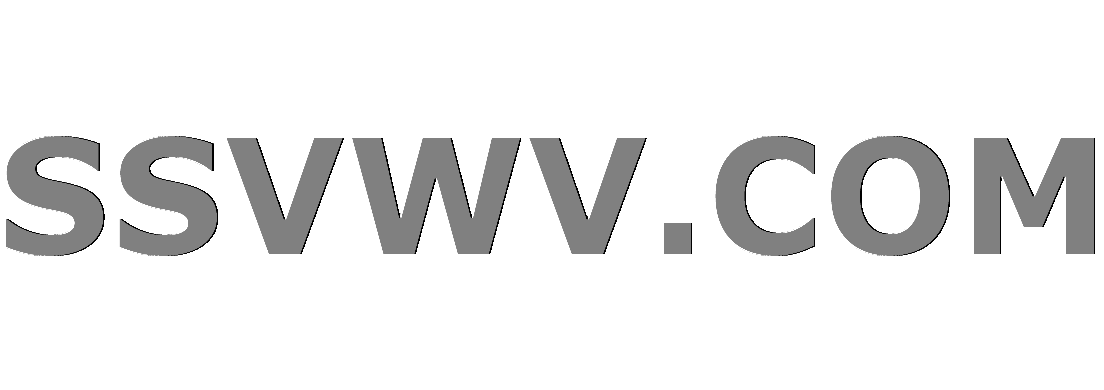
Multi tool use
up vote
0
down vote
favorite
I have created a simple question apps, when clicked on questions it shows its options or choices.I made login and signup forms to make user-login.
I want to know how can I limit the user to delete the questions created only by them.Every question has a delete key infront of it.
I read most stuff about permissions but didnt got it how to do it.
I may apply permission not to delete any question but how to restrict a user not to delete only some specific questions or questions that weren't created by them.
below is views.py
def addquestion(request):
item_to_add = request.POST['content']
item = Question.objects.create(question_text=item_to_add,pub_date=timezone.now())
user_now = Question(user = request.user)
item.save()
return HttpResponseRedirect('/home/questions')
def deletequestion(request,question_id):
item_to_delete = Question.objects.get(id=question_id)
if item_to_delete.user == request.user:
item_to_delete.delete()
else:
return HttpResponse('You are not authorised to delete this question')
Here is models .py
from django.db import models
from vote.models import VoteModel
from django.contrib.auth.models import User
# Create your models here.
class Question(VoteModel,models.Model):
question_text = models.TextField(max_length=300)
pub_date = models.DateTimeField('date published')
user = models.OneToOneField(User,on_delete = models.CASCADE,null=True)
def __str__(self):
return self.question_text
class Choice(models.Model):
choice_text = models.CharField(max_length=300)
votes = models.IntegerField(default=0)
question = models.ForeignKey(Question,on_delete = models.CASCADE)
def __str__(self):
return self.choice_text
python django authentication view web-development-server
add a comment |
up vote
0
down vote
favorite
I have created a simple question apps, when clicked on questions it shows its options or choices.I made login and signup forms to make user-login.
I want to know how can I limit the user to delete the questions created only by them.Every question has a delete key infront of it.
I read most stuff about permissions but didnt got it how to do it.
I may apply permission not to delete any question but how to restrict a user not to delete only some specific questions or questions that weren't created by them.
below is views.py
def addquestion(request):
item_to_add = request.POST['content']
item = Question.objects.create(question_text=item_to_add,pub_date=timezone.now())
user_now = Question(user = request.user)
item.save()
return HttpResponseRedirect('/home/questions')
def deletequestion(request,question_id):
item_to_delete = Question.objects.get(id=question_id)
if item_to_delete.user == request.user:
item_to_delete.delete()
else:
return HttpResponse('You are not authorised to delete this question')
Here is models .py
from django.db import models
from vote.models import VoteModel
from django.contrib.auth.models import User
# Create your models here.
class Question(VoteModel,models.Model):
question_text = models.TextField(max_length=300)
pub_date = models.DateTimeField('date published')
user = models.OneToOneField(User,on_delete = models.CASCADE,null=True)
def __str__(self):
return self.question_text
class Choice(models.Model):
choice_text = models.CharField(max_length=300)
votes = models.IntegerField(default=0)
question = models.ForeignKey(Question,on_delete = models.CASCADE)
def __str__(self):
return self.choice_text
python django authentication view web-development-server
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have created a simple question apps, when clicked on questions it shows its options or choices.I made login and signup forms to make user-login.
I want to know how can I limit the user to delete the questions created only by them.Every question has a delete key infront of it.
I read most stuff about permissions but didnt got it how to do it.
I may apply permission not to delete any question but how to restrict a user not to delete only some specific questions or questions that weren't created by them.
below is views.py
def addquestion(request):
item_to_add = request.POST['content']
item = Question.objects.create(question_text=item_to_add,pub_date=timezone.now())
user_now = Question(user = request.user)
item.save()
return HttpResponseRedirect('/home/questions')
def deletequestion(request,question_id):
item_to_delete = Question.objects.get(id=question_id)
if item_to_delete.user == request.user:
item_to_delete.delete()
else:
return HttpResponse('You are not authorised to delete this question')
Here is models .py
from django.db import models
from vote.models import VoteModel
from django.contrib.auth.models import User
# Create your models here.
class Question(VoteModel,models.Model):
question_text = models.TextField(max_length=300)
pub_date = models.DateTimeField('date published')
user = models.OneToOneField(User,on_delete = models.CASCADE,null=True)
def __str__(self):
return self.question_text
class Choice(models.Model):
choice_text = models.CharField(max_length=300)
votes = models.IntegerField(default=0)
question = models.ForeignKey(Question,on_delete = models.CASCADE)
def __str__(self):
return self.choice_text
python django authentication view web-development-server
I have created a simple question apps, when clicked on questions it shows its options or choices.I made login and signup forms to make user-login.
I want to know how can I limit the user to delete the questions created only by them.Every question has a delete key infront of it.
I read most stuff about permissions but didnt got it how to do it.
I may apply permission not to delete any question but how to restrict a user not to delete only some specific questions or questions that weren't created by them.
below is views.py
def addquestion(request):
item_to_add = request.POST['content']
item = Question.objects.create(question_text=item_to_add,pub_date=timezone.now())
user_now = Question(user = request.user)
item.save()
return HttpResponseRedirect('/home/questions')
def deletequestion(request,question_id):
item_to_delete = Question.objects.get(id=question_id)
if item_to_delete.user == request.user:
item_to_delete.delete()
else:
return HttpResponse('You are not authorised to delete this question')
Here is models .py
from django.db import models
from vote.models import VoteModel
from django.contrib.auth.models import User
# Create your models here.
class Question(VoteModel,models.Model):
question_text = models.TextField(max_length=300)
pub_date = models.DateTimeField('date published')
user = models.OneToOneField(User,on_delete = models.CASCADE,null=True)
def __str__(self):
return self.question_text
class Choice(models.Model):
choice_text = models.CharField(max_length=300)
votes = models.IntegerField(default=0)
question = models.ForeignKey(Question,on_delete = models.CASCADE)
def __str__(self):
return self.choice_text
python django authentication view web-development-server
python django authentication view web-development-server
edited Nov 10 at 23:35
Andrea Corbellini
12.2k13346
12.2k13346
asked Nov 10 at 21:37
Jidnyesh
82
82
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
accepted
You should filter your questions queryset:
from django.db.models import Q
...
try:
Question.objects.get(Q(id=question_id)&Q(user=request.user)).delete()
except Question.DoesNotExist:
raise PermissionDenied("User can't delete this question.")
...
The Q object allows you to logically operate with filters.
Reference: https://docs.djangoproject.com/es/2.1/topics/db/queries/#complex-lookups-with-q-objects
Update:
As pointed out in comments, in this particular case you can achieve this by doing the following:
try:
Question.objects.get(id=question_id, user=request.user).delete()
except Question.DoesNotExist:
raise PermissionDenied("User can't delete this question.")
get(Q(id=question_id)&Q(user=request.user))
is the same asget(id=question_id, user=request.user)
, just longer
– Andrea Corbellini
Nov 10 at 23:36
what is your issue right now?
– Juan Ignacio Sánchez
Nov 11 at 6:08
Into the deletequestion view , I did what u explained , I am creating a question , its also appearing on the question page , But when I click on delete option, its responding a permission denied error even though that question was created by me.
– Jidnyesh
Nov 11 at 6:09
I did and it worked but the problem is when i set the user field to user instance , it dont let me create multiple questions , i can only created one question.
– Jidnyesh
Nov 11 at 6:17
that is because you use a OneToOneField, change it to a ForeignKeyField
– Juan Ignacio Sánchez
Nov 11 at 6:19
|
show 4 more comments
up vote
0
down vote
Maybe request.user is a string that contains the id of the user so you will have to get the user object of that id before comparing it with item_to_dele.user.
logged_user = User.objects.get(id=request.user)
if logged_user == item_to_delete.user:
# delete
item_to_delete.delete()
else:
return HttpResponse('You are not authorised to delete this question')
request.user
should already be aUser
object: there's no need forUser.objects.get(id=request.user)
(unless the authentication system has been customized). Also, it'd be better to raise aPermissionDenied
, so that the user will get a 401 response instead of 200
– Andrea Corbellini
Nov 10 at 23:38
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You should filter your questions queryset:
from django.db.models import Q
...
try:
Question.objects.get(Q(id=question_id)&Q(user=request.user)).delete()
except Question.DoesNotExist:
raise PermissionDenied("User can't delete this question.")
...
The Q object allows you to logically operate with filters.
Reference: https://docs.djangoproject.com/es/2.1/topics/db/queries/#complex-lookups-with-q-objects
Update:
As pointed out in comments, in this particular case you can achieve this by doing the following:
try:
Question.objects.get(id=question_id, user=request.user).delete()
except Question.DoesNotExist:
raise PermissionDenied("User can't delete this question.")
get(Q(id=question_id)&Q(user=request.user))
is the same asget(id=question_id, user=request.user)
, just longer
– Andrea Corbellini
Nov 10 at 23:36
what is your issue right now?
– Juan Ignacio Sánchez
Nov 11 at 6:08
Into the deletequestion view , I did what u explained , I am creating a question , its also appearing on the question page , But when I click on delete option, its responding a permission denied error even though that question was created by me.
– Jidnyesh
Nov 11 at 6:09
I did and it worked but the problem is when i set the user field to user instance , it dont let me create multiple questions , i can only created one question.
– Jidnyesh
Nov 11 at 6:17
that is because you use a OneToOneField, change it to a ForeignKeyField
– Juan Ignacio Sánchez
Nov 11 at 6:19
|
show 4 more comments
up vote
1
down vote
accepted
You should filter your questions queryset:
from django.db.models import Q
...
try:
Question.objects.get(Q(id=question_id)&Q(user=request.user)).delete()
except Question.DoesNotExist:
raise PermissionDenied("User can't delete this question.")
...
The Q object allows you to logically operate with filters.
Reference: https://docs.djangoproject.com/es/2.1/topics/db/queries/#complex-lookups-with-q-objects
Update:
As pointed out in comments, in this particular case you can achieve this by doing the following:
try:
Question.objects.get(id=question_id, user=request.user).delete()
except Question.DoesNotExist:
raise PermissionDenied("User can't delete this question.")
get(Q(id=question_id)&Q(user=request.user))
is the same asget(id=question_id, user=request.user)
, just longer
– Andrea Corbellini
Nov 10 at 23:36
what is your issue right now?
– Juan Ignacio Sánchez
Nov 11 at 6:08
Into the deletequestion view , I did what u explained , I am creating a question , its also appearing on the question page , But when I click on delete option, its responding a permission denied error even though that question was created by me.
– Jidnyesh
Nov 11 at 6:09
I did and it worked but the problem is when i set the user field to user instance , it dont let me create multiple questions , i can only created one question.
– Jidnyesh
Nov 11 at 6:17
that is because you use a OneToOneField, change it to a ForeignKeyField
– Juan Ignacio Sánchez
Nov 11 at 6:19
|
show 4 more comments
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You should filter your questions queryset:
from django.db.models import Q
...
try:
Question.objects.get(Q(id=question_id)&Q(user=request.user)).delete()
except Question.DoesNotExist:
raise PermissionDenied("User can't delete this question.")
...
The Q object allows you to logically operate with filters.
Reference: https://docs.djangoproject.com/es/2.1/topics/db/queries/#complex-lookups-with-q-objects
Update:
As pointed out in comments, in this particular case you can achieve this by doing the following:
try:
Question.objects.get(id=question_id, user=request.user).delete()
except Question.DoesNotExist:
raise PermissionDenied("User can't delete this question.")
You should filter your questions queryset:
from django.db.models import Q
...
try:
Question.objects.get(Q(id=question_id)&Q(user=request.user)).delete()
except Question.DoesNotExist:
raise PermissionDenied("User can't delete this question.")
...
The Q object allows you to logically operate with filters.
Reference: https://docs.djangoproject.com/es/2.1/topics/db/queries/#complex-lookups-with-q-objects
Update:
As pointed out in comments, in this particular case you can achieve this by doing the following:
try:
Question.objects.get(id=question_id, user=request.user).delete()
except Question.DoesNotExist:
raise PermissionDenied("User can't delete this question.")
edited Nov 11 at 0:00
answered Nov 10 at 23:15


Juan Ignacio Sánchez
305111
305111
get(Q(id=question_id)&Q(user=request.user))
is the same asget(id=question_id, user=request.user)
, just longer
– Andrea Corbellini
Nov 10 at 23:36
what is your issue right now?
– Juan Ignacio Sánchez
Nov 11 at 6:08
Into the deletequestion view , I did what u explained , I am creating a question , its also appearing on the question page , But when I click on delete option, its responding a permission denied error even though that question was created by me.
– Jidnyesh
Nov 11 at 6:09
I did and it worked but the problem is when i set the user field to user instance , it dont let me create multiple questions , i can only created one question.
– Jidnyesh
Nov 11 at 6:17
that is because you use a OneToOneField, change it to a ForeignKeyField
– Juan Ignacio Sánchez
Nov 11 at 6:19
|
show 4 more comments
get(Q(id=question_id)&Q(user=request.user))
is the same asget(id=question_id, user=request.user)
, just longer
– Andrea Corbellini
Nov 10 at 23:36
what is your issue right now?
– Juan Ignacio Sánchez
Nov 11 at 6:08
Into the deletequestion view , I did what u explained , I am creating a question , its also appearing on the question page , But when I click on delete option, its responding a permission denied error even though that question was created by me.
– Jidnyesh
Nov 11 at 6:09
I did and it worked but the problem is when i set the user field to user instance , it dont let me create multiple questions , i can only created one question.
– Jidnyesh
Nov 11 at 6:17
that is because you use a OneToOneField, change it to a ForeignKeyField
– Juan Ignacio Sánchez
Nov 11 at 6:19
get(Q(id=question_id)&Q(user=request.user))
is the same as get(id=question_id, user=request.user)
, just longer– Andrea Corbellini
Nov 10 at 23:36
get(Q(id=question_id)&Q(user=request.user))
is the same as get(id=question_id, user=request.user)
, just longer– Andrea Corbellini
Nov 10 at 23:36
what is your issue right now?
– Juan Ignacio Sánchez
Nov 11 at 6:08
what is your issue right now?
– Juan Ignacio Sánchez
Nov 11 at 6:08
Into the deletequestion view , I did what u explained , I am creating a question , its also appearing on the question page , But when I click on delete option, its responding a permission denied error even though that question was created by me.
– Jidnyesh
Nov 11 at 6:09
Into the deletequestion view , I did what u explained , I am creating a question , its also appearing on the question page , But when I click on delete option, its responding a permission denied error even though that question was created by me.
– Jidnyesh
Nov 11 at 6:09
I did and it worked but the problem is when i set the user field to user instance , it dont let me create multiple questions , i can only created one question.
– Jidnyesh
Nov 11 at 6:17
I did and it worked but the problem is when i set the user field to user instance , it dont let me create multiple questions , i can only created one question.
– Jidnyesh
Nov 11 at 6:17
that is because you use a OneToOneField, change it to a ForeignKeyField
– Juan Ignacio Sánchez
Nov 11 at 6:19
that is because you use a OneToOneField, change it to a ForeignKeyField
– Juan Ignacio Sánchez
Nov 11 at 6:19
|
show 4 more comments
up vote
0
down vote
Maybe request.user is a string that contains the id of the user so you will have to get the user object of that id before comparing it with item_to_dele.user.
logged_user = User.objects.get(id=request.user)
if logged_user == item_to_delete.user:
# delete
item_to_delete.delete()
else:
return HttpResponse('You are not authorised to delete this question')
request.user
should already be aUser
object: there's no need forUser.objects.get(id=request.user)
(unless the authentication system has been customized). Also, it'd be better to raise aPermissionDenied
, so that the user will get a 401 response instead of 200
– Andrea Corbellini
Nov 10 at 23:38
add a comment |
up vote
0
down vote
Maybe request.user is a string that contains the id of the user so you will have to get the user object of that id before comparing it with item_to_dele.user.
logged_user = User.objects.get(id=request.user)
if logged_user == item_to_delete.user:
# delete
item_to_delete.delete()
else:
return HttpResponse('You are not authorised to delete this question')
request.user
should already be aUser
object: there's no need forUser.objects.get(id=request.user)
(unless the authentication system has been customized). Also, it'd be better to raise aPermissionDenied
, so that the user will get a 401 response instead of 200
– Andrea Corbellini
Nov 10 at 23:38
add a comment |
up vote
0
down vote
up vote
0
down vote
Maybe request.user is a string that contains the id of the user so you will have to get the user object of that id before comparing it with item_to_dele.user.
logged_user = User.objects.get(id=request.user)
if logged_user == item_to_delete.user:
# delete
item_to_delete.delete()
else:
return HttpResponse('You are not authorised to delete this question')
Maybe request.user is a string that contains the id of the user so you will have to get the user object of that id before comparing it with item_to_dele.user.
logged_user = User.objects.get(id=request.user)
if logged_user == item_to_delete.user:
# delete
item_to_delete.delete()
else:
return HttpResponse('You are not authorised to delete this question')
answered Nov 10 at 23:15
Amine Messaoudi
182113
182113
request.user
should already be aUser
object: there's no need forUser.objects.get(id=request.user)
(unless the authentication system has been customized). Also, it'd be better to raise aPermissionDenied
, so that the user will get a 401 response instead of 200
– Andrea Corbellini
Nov 10 at 23:38
add a comment |
request.user
should already be aUser
object: there's no need forUser.objects.get(id=request.user)
(unless the authentication system has been customized). Also, it'd be better to raise aPermissionDenied
, so that the user will get a 401 response instead of 200
– Andrea Corbellini
Nov 10 at 23:38
request.user
should already be a User
object: there's no need for User.objects.get(id=request.user)
(unless the authentication system has been customized). Also, it'd be better to raise a PermissionDenied
, so that the user will get a 401 response instead of 200– Andrea Corbellini
Nov 10 at 23:38
request.user
should already be a User
object: there's no need for User.objects.get(id=request.user)
(unless the authentication system has been customized). Also, it'd be better to raise a PermissionDenied
, so that the user will get a 401 response instead of 200– Andrea Corbellini
Nov 10 at 23:38
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53243647%2fin-a-django-app-how-do-i-prevent-users-from-deleting-content-not-created-by-the%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
YvKhjnHQxcsR CE6ffT5FL5zwaBxfx u,pKz S