how to send user latitude and longitude to django view
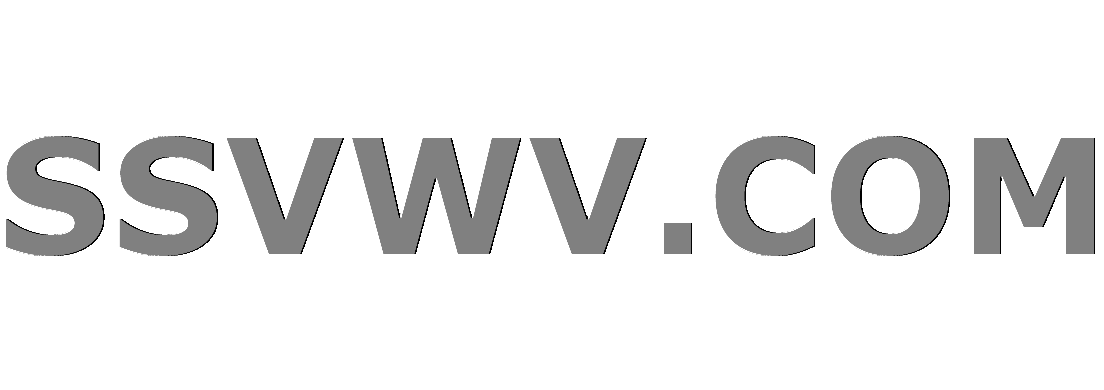
Multi tool use
up vote
-1
down vote
favorite
This is the javascript code to get users latitude and longitude.
<!DOCTYPE html>
<html>
<body>
<p>Click the button to get your coordinates.</p>
<button onclick="getLocation()">Try It</button>
<p id="demo"></p>
<script>
var x = document.getElementById("demo");
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
} else {
x.innerHTML = "Geolocation is not supported by this browser.";
}
}
function showPosition(position) {
lat = position.coords.latitude;
long = position.coords.longitude;
x.innerHTML = "Latitude: " +lat +
"<br>Longitude: " +long ;
}
</script>
</body>
</html>
through that code, I can display the user's latitude and longitude in the front end, but I need to send the lat and long to Django views.py to show the nearby local business. how can i do that?
i've went through this solution but i'm unable to do that. can anyone help me?
EDIT
i have added ajax to code still not able to send lat value to the views.py
<!DOCTYPE html>
<html>
<body>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
<p>Click the button to get your coordinates.</p>
<button id="send-my-url-to-django-button" onclick="getLocation()">Try It</button>
<p id="demo"></p>
<script>
var x = document.getElementById("demo");
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
} else {
x.innerHTML = "Geolocation is not supported by this browser.";
}
}
function showPosition(position) {
lat = position.coords.latitude;
long = position.coords.longitude;
x.innerHTML = "Latitude: " +lat +
"<br>Longitude: " +long ;
$(document).on('click',function() {
var lat1 = lat;
var long1 = long;
$("#send-my-url-to-django-button").click(function() {
$.ajax({
url: '{% url "accounts:location" %}',
//type: 'POST',
contentType: 'application/json; charset=utf-8',
dataType: "json",
data: {
'lat': lat1,
'long': long1,
//'csrfmiddlewaretoken': '{{ csrf_token }}'
},
success : function(json) {
alert("Successfully sent the URL to Django");
},
error : function(xhr,errmsg,err) {
alert("Could not send URL to Django. Error: " + xhr.status + ": " + xhr.responseText);
}
});
});
});
}
</script>
</body>
</html
after clicking 3 times, i receive lat ang long values in views.py and in browser it shows
Could not send URL to django Error:200;
javascript django-views
add a comment |
up vote
-1
down vote
favorite
This is the javascript code to get users latitude and longitude.
<!DOCTYPE html>
<html>
<body>
<p>Click the button to get your coordinates.</p>
<button onclick="getLocation()">Try It</button>
<p id="demo"></p>
<script>
var x = document.getElementById("demo");
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
} else {
x.innerHTML = "Geolocation is not supported by this browser.";
}
}
function showPosition(position) {
lat = position.coords.latitude;
long = position.coords.longitude;
x.innerHTML = "Latitude: " +lat +
"<br>Longitude: " +long ;
}
</script>
</body>
</html>
through that code, I can display the user's latitude and longitude in the front end, but I need to send the lat and long to Django views.py to show the nearby local business. how can i do that?
i've went through this solution but i'm unable to do that. can anyone help me?
EDIT
i have added ajax to code still not able to send lat value to the views.py
<!DOCTYPE html>
<html>
<body>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
<p>Click the button to get your coordinates.</p>
<button id="send-my-url-to-django-button" onclick="getLocation()">Try It</button>
<p id="demo"></p>
<script>
var x = document.getElementById("demo");
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
} else {
x.innerHTML = "Geolocation is not supported by this browser.";
}
}
function showPosition(position) {
lat = position.coords.latitude;
long = position.coords.longitude;
x.innerHTML = "Latitude: " +lat +
"<br>Longitude: " +long ;
$(document).on('click',function() {
var lat1 = lat;
var long1 = long;
$("#send-my-url-to-django-button").click(function() {
$.ajax({
url: '{% url "accounts:location" %}',
//type: 'POST',
contentType: 'application/json; charset=utf-8',
dataType: "json",
data: {
'lat': lat1,
'long': long1,
//'csrfmiddlewaretoken': '{{ csrf_token }}'
},
success : function(json) {
alert("Successfully sent the URL to Django");
},
error : function(xhr,errmsg,err) {
alert("Could not send URL to Django. Error: " + xhr.status + ": " + xhr.responseText);
}
});
});
});
}
</script>
</body>
</html
after clicking 3 times, i receive lat ang long values in views.py and in browser it shows
Could not send URL to django Error:200;
javascript django-views
Have you tried HTTP? You know, that thing between your server and your browser.
– sjahan
Nov 11 at 8:16
HTTP means send data using POST and GET methods?
– Prince Nihith
Nov 11 at 8:32
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
This is the javascript code to get users latitude and longitude.
<!DOCTYPE html>
<html>
<body>
<p>Click the button to get your coordinates.</p>
<button onclick="getLocation()">Try It</button>
<p id="demo"></p>
<script>
var x = document.getElementById("demo");
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
} else {
x.innerHTML = "Geolocation is not supported by this browser.";
}
}
function showPosition(position) {
lat = position.coords.latitude;
long = position.coords.longitude;
x.innerHTML = "Latitude: " +lat +
"<br>Longitude: " +long ;
}
</script>
</body>
</html>
through that code, I can display the user's latitude and longitude in the front end, but I need to send the lat and long to Django views.py to show the nearby local business. how can i do that?
i've went through this solution but i'm unable to do that. can anyone help me?
EDIT
i have added ajax to code still not able to send lat value to the views.py
<!DOCTYPE html>
<html>
<body>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
<p>Click the button to get your coordinates.</p>
<button id="send-my-url-to-django-button" onclick="getLocation()">Try It</button>
<p id="demo"></p>
<script>
var x = document.getElementById("demo");
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
} else {
x.innerHTML = "Geolocation is not supported by this browser.";
}
}
function showPosition(position) {
lat = position.coords.latitude;
long = position.coords.longitude;
x.innerHTML = "Latitude: " +lat +
"<br>Longitude: " +long ;
$(document).on('click',function() {
var lat1 = lat;
var long1 = long;
$("#send-my-url-to-django-button").click(function() {
$.ajax({
url: '{% url "accounts:location" %}',
//type: 'POST',
contentType: 'application/json; charset=utf-8',
dataType: "json",
data: {
'lat': lat1,
'long': long1,
//'csrfmiddlewaretoken': '{{ csrf_token }}'
},
success : function(json) {
alert("Successfully sent the URL to Django");
},
error : function(xhr,errmsg,err) {
alert("Could not send URL to Django. Error: " + xhr.status + ": " + xhr.responseText);
}
});
});
});
}
</script>
</body>
</html
after clicking 3 times, i receive lat ang long values in views.py and in browser it shows
Could not send URL to django Error:200;
javascript django-views
This is the javascript code to get users latitude and longitude.
<!DOCTYPE html>
<html>
<body>
<p>Click the button to get your coordinates.</p>
<button onclick="getLocation()">Try It</button>
<p id="demo"></p>
<script>
var x = document.getElementById("demo");
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
} else {
x.innerHTML = "Geolocation is not supported by this browser.";
}
}
function showPosition(position) {
lat = position.coords.latitude;
long = position.coords.longitude;
x.innerHTML = "Latitude: " +lat +
"<br>Longitude: " +long ;
}
</script>
</body>
</html>
through that code, I can display the user's latitude and longitude in the front end, but I need to send the lat and long to Django views.py to show the nearby local business. how can i do that?
i've went through this solution but i'm unable to do that. can anyone help me?
EDIT
i have added ajax to code still not able to send lat value to the views.py
<!DOCTYPE html>
<html>
<body>
<script src="http://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"></script>
<p>Click the button to get your coordinates.</p>
<button id="send-my-url-to-django-button" onclick="getLocation()">Try It</button>
<p id="demo"></p>
<script>
var x = document.getElementById("demo");
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
} else {
x.innerHTML = "Geolocation is not supported by this browser.";
}
}
function showPosition(position) {
lat = position.coords.latitude;
long = position.coords.longitude;
x.innerHTML = "Latitude: " +lat +
"<br>Longitude: " +long ;
$(document).on('click',function() {
var lat1 = lat;
var long1 = long;
$("#send-my-url-to-django-button").click(function() {
$.ajax({
url: '{% url "accounts:location" %}',
//type: 'POST',
contentType: 'application/json; charset=utf-8',
dataType: "json",
data: {
'lat': lat1,
'long': long1,
//'csrfmiddlewaretoken': '{{ csrf_token }}'
},
success : function(json) {
alert("Successfully sent the URL to Django");
},
error : function(xhr,errmsg,err) {
alert("Could not send URL to Django. Error: " + xhr.status + ": " + xhr.responseText);
}
});
});
});
}
</script>
</body>
</html
after clicking 3 times, i receive lat ang long values in views.py and in browser it shows
Could not send URL to django Error:200;
javascript django-views
javascript django-views
edited Nov 11 at 9:46
asked Nov 11 at 7:47
Prince Nihith
74
74
Have you tried HTTP? You know, that thing between your server and your browser.
– sjahan
Nov 11 at 8:16
HTTP means send data using POST and GET methods?
– Prince Nihith
Nov 11 at 8:32
add a comment |
Have you tried HTTP? You know, that thing between your server and your browser.
– sjahan
Nov 11 at 8:16
HTTP means send data using POST and GET methods?
– Prince Nihith
Nov 11 at 8:32
Have you tried HTTP? You know, that thing between your server and your browser.
– sjahan
Nov 11 at 8:16
Have you tried HTTP? You know, that thing between your server and your browser.
– sjahan
Nov 11 at 8:16
HTTP means send data using POST and GET methods?
– Prince Nihith
Nov 11 at 8:32
HTTP means send data using POST and GET methods?
– Prince Nihith
Nov 11 at 8:32
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53246800%2fhow-to-send-user-latitude-and-longitude-to-django-view%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Yo8wi lWIT4Va,092mvW1HT1R31boynymBDmdc h0kc
Have you tried HTTP? You know, that thing between your server and your browser.
– sjahan
Nov 11 at 8:16
HTTP means send data using POST and GET methods?
– Prince Nihith
Nov 11 at 8:32