Dynamically setting Padding widget so Column is not covered by FAB
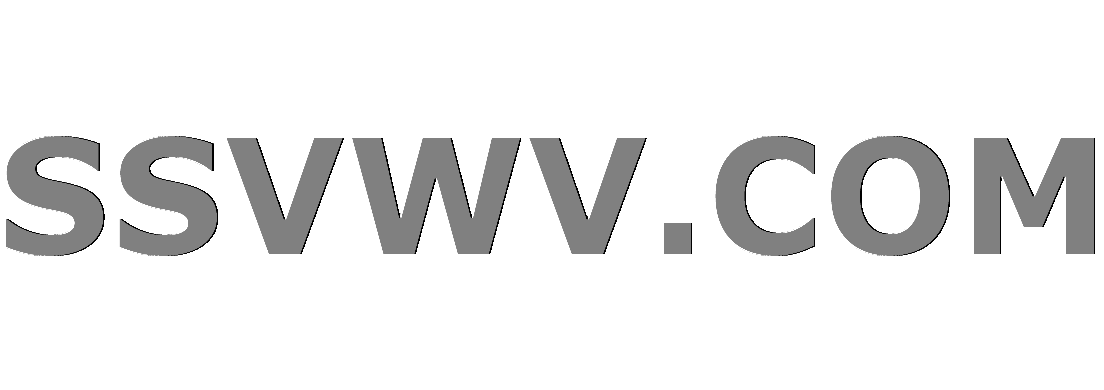
Multi tool use
Is there a way to dynamically add bottom padding to a Column
so it is never obscured by the FAB?
For example, this has text covered by the FAB.
I add 50
amount of padding with Container(child: Padding(padding: EdgeInsets.all(50.0)),)
so it is no longer covered and now looks like this:
My question is there a way to dynamically set this based on the height of the FAB? In my case the FAB is a button, but the FAB can be any widget of any height. So I want to avoid the 50.0
constant.
This is all the code used in the demo:
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
home: View(),
);
}
}
class View extends StatelessWidget {
final paragraphText = 'Example of a paragraph of text.' * 100;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: new AppBar(),
floatingActionButton: FloatingActionButton(
onPressed: () {},
child: Icon(Icons.add),
),
body: SingleChildScrollView(
child: Column(
children: <Widget>[
MaterialButton(onPressed: (){}, child: Text('Button example'),),
Text(paragraphText),
Container(child: Padding(padding: EdgeInsets.all(50.0)),)
],
),
),
);
}
}
dart

add a comment |
Is there a way to dynamically add bottom padding to a Column
so it is never obscured by the FAB?
For example, this has text covered by the FAB.
I add 50
amount of padding with Container(child: Padding(padding: EdgeInsets.all(50.0)),)
so it is no longer covered and now looks like this:
My question is there a way to dynamically set this based on the height of the FAB? In my case the FAB is a button, but the FAB can be any widget of any height. So I want to avoid the 50.0
constant.
This is all the code used in the demo:
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
home: View(),
);
}
}
class View extends StatelessWidget {
final paragraphText = 'Example of a paragraph of text.' * 100;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: new AppBar(),
floatingActionButton: FloatingActionButton(
onPressed: () {},
child: Icon(Icons.add),
),
body: SingleChildScrollView(
child: Column(
children: <Widget>[
MaterialButton(onPressed: (){}, child: Text('Button example'),),
Text(paragraphText),
Container(child: Padding(padding: EdgeInsets.all(50.0)),)
],
),
),
);
}
}
dart

add a comment |
Is there a way to dynamically add bottom padding to a Column
so it is never obscured by the FAB?
For example, this has text covered by the FAB.
I add 50
amount of padding with Container(child: Padding(padding: EdgeInsets.all(50.0)),)
so it is no longer covered and now looks like this:
My question is there a way to dynamically set this based on the height of the FAB? In my case the FAB is a button, but the FAB can be any widget of any height. So I want to avoid the 50.0
constant.
This is all the code used in the demo:
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
home: View(),
);
}
}
class View extends StatelessWidget {
final paragraphText = 'Example of a paragraph of text.' * 100;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: new AppBar(),
floatingActionButton: FloatingActionButton(
onPressed: () {},
child: Icon(Icons.add),
),
body: SingleChildScrollView(
child: Column(
children: <Widget>[
MaterialButton(onPressed: (){}, child: Text('Button example'),),
Text(paragraphText),
Container(child: Padding(padding: EdgeInsets.all(50.0)),)
],
),
),
);
}
}
dart

Is there a way to dynamically add bottom padding to a Column
so it is never obscured by the FAB?
For example, this has text covered by the FAB.
I add 50
amount of padding with Container(child: Padding(padding: EdgeInsets.all(50.0)),)
so it is no longer covered and now looks like this:
My question is there a way to dynamically set this based on the height of the FAB? In my case the FAB is a button, but the FAB can be any widget of any height. So I want to avoid the 50.0
constant.
This is all the code used in the demo:
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
home: View(),
);
}
}
class View extends StatelessWidget {
final paragraphText = 'Example of a paragraph of text.' * 100;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: new AppBar(),
floatingActionButton: FloatingActionButton(
onPressed: () {},
child: Icon(Icons.add),
),
body: SingleChildScrollView(
child: Column(
children: <Widget>[
MaterialButton(onPressed: (){}, child: Text('Button example'),),
Text(paragraphText),
Container(child: Padding(padding: EdgeInsets.all(50.0)),)
],
),
),
);
}
}
dart

dart

asked Nov 13 '18 at 22:09
S.D.S.D.
202113
202113
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You can do the following:
- assign a
GlobalKey
to your fab widget. - wait after the frame is loaded.
- get the size of the fab widget using the
GlobalKey
andRenderBox
. - declare a variable to store the padding.
- call
setState
with the padding updated.
```
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
home: View(),
);
}
}
class View extends StatefulWidget {
@override
ViewState createState() {
return new ViewState();
}
}
class ViewState extends State<View> {
final paragraphText = 'Example of a paragraph of text.' * 100;
GlobalKey _keyFab = GlobalKey();
double padding = 0.0;
_onLayoutDone(_) {
RenderBox renderObject = _keyFab.currentContext.findRenderObject();
setState(() {
padding = renderObject.size.height;
});
print("padding: $padding");
}
@override
void initState() {
WidgetsBinding.instance.addPostFrameCallback(_onLayoutDone);
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: new AppBar(),
floatingActionButton: FloatingActionButton(
key: _keyFab,
onPressed: () {},
child: Icon(Icons.add),
),
body: SingleChildScrollView(
child: Column(
children: <Widget>[
MaterialButton(
onPressed: () {},
child: Text('Button example'),
),
Text(paragraphText),
Container(
child: Padding(padding: EdgeInsets.all(padding)),
)
],
),
),
);
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53290253%2fdynamically-setting-padding-widget-so-column-is-not-covered-by-fab%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can do the following:
- assign a
GlobalKey
to your fab widget. - wait after the frame is loaded.
- get the size of the fab widget using the
GlobalKey
andRenderBox
. - declare a variable to store the padding.
- call
setState
with the padding updated.
```
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
home: View(),
);
}
}
class View extends StatefulWidget {
@override
ViewState createState() {
return new ViewState();
}
}
class ViewState extends State<View> {
final paragraphText = 'Example of a paragraph of text.' * 100;
GlobalKey _keyFab = GlobalKey();
double padding = 0.0;
_onLayoutDone(_) {
RenderBox renderObject = _keyFab.currentContext.findRenderObject();
setState(() {
padding = renderObject.size.height;
});
print("padding: $padding");
}
@override
void initState() {
WidgetsBinding.instance.addPostFrameCallback(_onLayoutDone);
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: new AppBar(),
floatingActionButton: FloatingActionButton(
key: _keyFab,
onPressed: () {},
child: Icon(Icons.add),
),
body: SingleChildScrollView(
child: Column(
children: <Widget>[
MaterialButton(
onPressed: () {},
child: Text('Button example'),
),
Text(paragraphText),
Container(
child: Padding(padding: EdgeInsets.all(padding)),
)
],
),
),
);
}
}
add a comment |
You can do the following:
- assign a
GlobalKey
to your fab widget. - wait after the frame is loaded.
- get the size of the fab widget using the
GlobalKey
andRenderBox
. - declare a variable to store the padding.
- call
setState
with the padding updated.
```
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
home: View(),
);
}
}
class View extends StatefulWidget {
@override
ViewState createState() {
return new ViewState();
}
}
class ViewState extends State<View> {
final paragraphText = 'Example of a paragraph of text.' * 100;
GlobalKey _keyFab = GlobalKey();
double padding = 0.0;
_onLayoutDone(_) {
RenderBox renderObject = _keyFab.currentContext.findRenderObject();
setState(() {
padding = renderObject.size.height;
});
print("padding: $padding");
}
@override
void initState() {
WidgetsBinding.instance.addPostFrameCallback(_onLayoutDone);
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: new AppBar(),
floatingActionButton: FloatingActionButton(
key: _keyFab,
onPressed: () {},
child: Icon(Icons.add),
),
body: SingleChildScrollView(
child: Column(
children: <Widget>[
MaterialButton(
onPressed: () {},
child: Text('Button example'),
),
Text(paragraphText),
Container(
child: Padding(padding: EdgeInsets.all(padding)),
)
],
),
),
);
}
}
add a comment |
You can do the following:
- assign a
GlobalKey
to your fab widget. - wait after the frame is loaded.
- get the size of the fab widget using the
GlobalKey
andRenderBox
. - declare a variable to store the padding.
- call
setState
with the padding updated.
```
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
home: View(),
);
}
}
class View extends StatefulWidget {
@override
ViewState createState() {
return new ViewState();
}
}
class ViewState extends State<View> {
final paragraphText = 'Example of a paragraph of text.' * 100;
GlobalKey _keyFab = GlobalKey();
double padding = 0.0;
_onLayoutDone(_) {
RenderBox renderObject = _keyFab.currentContext.findRenderObject();
setState(() {
padding = renderObject.size.height;
});
print("padding: $padding");
}
@override
void initState() {
WidgetsBinding.instance.addPostFrameCallback(_onLayoutDone);
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: new AppBar(),
floatingActionButton: FloatingActionButton(
key: _keyFab,
onPressed: () {},
child: Icon(Icons.add),
),
body: SingleChildScrollView(
child: Column(
children: <Widget>[
MaterialButton(
onPressed: () {},
child: Text('Button example'),
),
Text(paragraphText),
Container(
child: Padding(padding: EdgeInsets.all(padding)),
)
],
),
),
);
}
}
You can do the following:
- assign a
GlobalKey
to your fab widget. - wait after the frame is loaded.
- get the size of the fab widget using the
GlobalKey
andRenderBox
. - declare a variable to store the padding.
- call
setState
with the padding updated.
```
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
home: View(),
);
}
}
class View extends StatefulWidget {
@override
ViewState createState() {
return new ViewState();
}
}
class ViewState extends State<View> {
final paragraphText = 'Example of a paragraph of text.' * 100;
GlobalKey _keyFab = GlobalKey();
double padding = 0.0;
_onLayoutDone(_) {
RenderBox renderObject = _keyFab.currentContext.findRenderObject();
setState(() {
padding = renderObject.size.height;
});
print("padding: $padding");
}
@override
void initState() {
WidgetsBinding.instance.addPostFrameCallback(_onLayoutDone);
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: new AppBar(),
floatingActionButton: FloatingActionButton(
key: _keyFab,
onPressed: () {},
child: Icon(Icons.add),
),
body: SingleChildScrollView(
child: Column(
children: <Widget>[
MaterialButton(
onPressed: () {},
child: Text('Button example'),
),
Text(paragraphText),
Container(
child: Padding(padding: EdgeInsets.all(padding)),
)
],
),
),
);
}
}
edited Nov 14 '18 at 14:03
answered Nov 14 '18 at 5:39


diegoveloperdiegoveloper
11.7k11630
11.7k11630
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53290253%2fdynamically-setting-padding-widget-so-column-is-not-covered-by-fab%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
NDIKBAa3gd,ISAVha3fjy,A,kRRlQ