Does Django's get_queryset() in admin prevent malicious object saving?
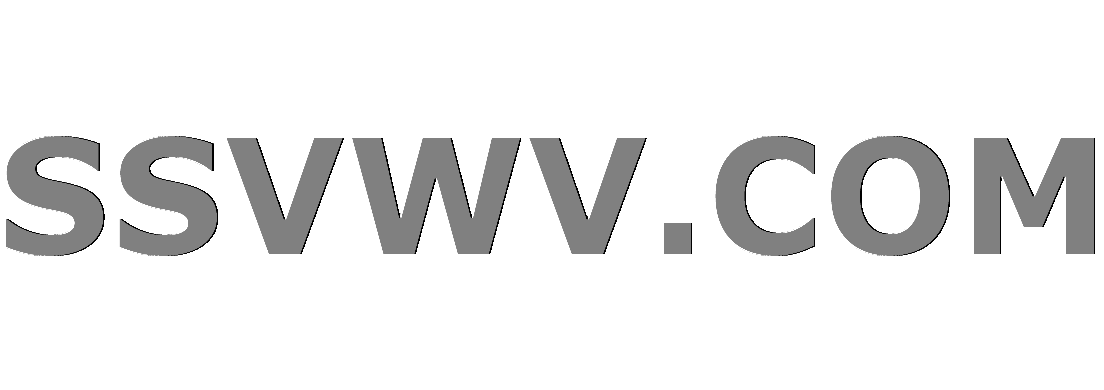
Multi tool use
I am developing a multi-tenant app in Django. In the Django admin, some querysets are filtered based on the user, using get_queryset()
.
Up till now, when a user updated an object from the Django change form, I would validate the data by creating a ModelAdmin
form using a factory function to capture the HttpRequest
object, then ensure that the Guest
object's user
was the current user:
EXAMPLE
models.py
class Guest(models.Model):
guest_name = models.CharField(max_length=64)
user = models.ForeignKey(User, on_delete=models.CASCADE)
admin.py
@admin.register(Guest)
class GuestAdmin(admin.ModelAdmin):
def get_queryset(self, request)
qs = super().get_queryset(request)
return qs.filter(user=request.user)
def get_form(self, request, obj=None, **kwargs):
self.form = _guest_admin_form_factory(request)
return super().get_form(request, obj, **kwargs)
forms.py
def _guest_admin_form_factory(request):
class GuestAdminForm(forms.ModelForm):
class Meta:
model = Guest
exclude =
def clean_user(self):
user = self.cleaned_data.get('user', None)
if not user:
return user
if user != request.user:
raise forms.ValidationError('Invalid request.')
return user
return GuestAdminForm
It occurred to me that Django might use the get_queryset()
method to validate this for me, since some simple logging showed that the method is called twice when an object gets updated from the change form.
Is this the case, or do I need to stick to validating through a ModelAdmin
form?
python django django-forms django-admin
add a comment |
I am developing a multi-tenant app in Django. In the Django admin, some querysets are filtered based on the user, using get_queryset()
.
Up till now, when a user updated an object from the Django change form, I would validate the data by creating a ModelAdmin
form using a factory function to capture the HttpRequest
object, then ensure that the Guest
object's user
was the current user:
EXAMPLE
models.py
class Guest(models.Model):
guest_name = models.CharField(max_length=64)
user = models.ForeignKey(User, on_delete=models.CASCADE)
admin.py
@admin.register(Guest)
class GuestAdmin(admin.ModelAdmin):
def get_queryset(self, request)
qs = super().get_queryset(request)
return qs.filter(user=request.user)
def get_form(self, request, obj=None, **kwargs):
self.form = _guest_admin_form_factory(request)
return super().get_form(request, obj, **kwargs)
forms.py
def _guest_admin_form_factory(request):
class GuestAdminForm(forms.ModelForm):
class Meta:
model = Guest
exclude =
def clean_user(self):
user = self.cleaned_data.get('user', None)
if not user:
return user
if user != request.user:
raise forms.ValidationError('Invalid request.')
return user
return GuestAdminForm
It occurred to me that Django might use the get_queryset()
method to validate this for me, since some simple logging showed that the method is called twice when an object gets updated from the change form.
Is this the case, or do I need to stick to validating through a ModelAdmin
form?
python django django-forms django-admin
Per Mr. Henry's answer, this is possible... but I would not call it advisable. It's a good idea to treat the Django admin as a low-level interface for top-level technical administration; if you have user-facing object manipulation, it's usually simpler and better to handle this with ModelForm or Form views and templates that can easily be altered/updated without worrying about what might change in the admin package, which is also a lot more complex to skin/alter, should those requirements come up. Particularly for multi-tenancy, I think this is a much more maintainable and debuggable approach.
– kungphu
Nov 13 at 5:47
add a comment |
I am developing a multi-tenant app in Django. In the Django admin, some querysets are filtered based on the user, using get_queryset()
.
Up till now, when a user updated an object from the Django change form, I would validate the data by creating a ModelAdmin
form using a factory function to capture the HttpRequest
object, then ensure that the Guest
object's user
was the current user:
EXAMPLE
models.py
class Guest(models.Model):
guest_name = models.CharField(max_length=64)
user = models.ForeignKey(User, on_delete=models.CASCADE)
admin.py
@admin.register(Guest)
class GuestAdmin(admin.ModelAdmin):
def get_queryset(self, request)
qs = super().get_queryset(request)
return qs.filter(user=request.user)
def get_form(self, request, obj=None, **kwargs):
self.form = _guest_admin_form_factory(request)
return super().get_form(request, obj, **kwargs)
forms.py
def _guest_admin_form_factory(request):
class GuestAdminForm(forms.ModelForm):
class Meta:
model = Guest
exclude =
def clean_user(self):
user = self.cleaned_data.get('user', None)
if not user:
return user
if user != request.user:
raise forms.ValidationError('Invalid request.')
return user
return GuestAdminForm
It occurred to me that Django might use the get_queryset()
method to validate this for me, since some simple logging showed that the method is called twice when an object gets updated from the change form.
Is this the case, or do I need to stick to validating through a ModelAdmin
form?
python django django-forms django-admin
I am developing a multi-tenant app in Django. In the Django admin, some querysets are filtered based on the user, using get_queryset()
.
Up till now, when a user updated an object from the Django change form, I would validate the data by creating a ModelAdmin
form using a factory function to capture the HttpRequest
object, then ensure that the Guest
object's user
was the current user:
EXAMPLE
models.py
class Guest(models.Model):
guest_name = models.CharField(max_length=64)
user = models.ForeignKey(User, on_delete=models.CASCADE)
admin.py
@admin.register(Guest)
class GuestAdmin(admin.ModelAdmin):
def get_queryset(self, request)
qs = super().get_queryset(request)
return qs.filter(user=request.user)
def get_form(self, request, obj=None, **kwargs):
self.form = _guest_admin_form_factory(request)
return super().get_form(request, obj, **kwargs)
forms.py
def _guest_admin_form_factory(request):
class GuestAdminForm(forms.ModelForm):
class Meta:
model = Guest
exclude =
def clean_user(self):
user = self.cleaned_data.get('user', None)
if not user:
return user
if user != request.user:
raise forms.ValidationError('Invalid request.')
return user
return GuestAdminForm
It occurred to me that Django might use the get_queryset()
method to validate this for me, since some simple logging showed that the method is called twice when an object gets updated from the change form.
Is this the case, or do I need to stick to validating through a ModelAdmin
form?
python django django-forms django-admin
python django django-forms django-admin
edited Nov 16 at 13:18
asked Nov 12 at 19:35
crazy4linux
615
615
Per Mr. Henry's answer, this is possible... but I would not call it advisable. It's a good idea to treat the Django admin as a low-level interface for top-level technical administration; if you have user-facing object manipulation, it's usually simpler and better to handle this with ModelForm or Form views and templates that can easily be altered/updated without worrying about what might change in the admin package, which is also a lot more complex to skin/alter, should those requirements come up. Particularly for multi-tenancy, I think this is a much more maintainable and debuggable approach.
– kungphu
Nov 13 at 5:47
add a comment |
Per Mr. Henry's answer, this is possible... but I would not call it advisable. It's a good idea to treat the Django admin as a low-level interface for top-level technical administration; if you have user-facing object manipulation, it's usually simpler and better to handle this with ModelForm or Form views and templates that can easily be altered/updated without worrying about what might change in the admin package, which is also a lot more complex to skin/alter, should those requirements come up. Particularly for multi-tenancy, I think this is a much more maintainable and debuggable approach.
– kungphu
Nov 13 at 5:47
Per Mr. Henry's answer, this is possible... but I would not call it advisable. It's a good idea to treat the Django admin as a low-level interface for top-level technical administration; if you have user-facing object manipulation, it's usually simpler and better to handle this with ModelForm or Form views and templates that can easily be altered/updated without worrying about what might change in the admin package, which is also a lot more complex to skin/alter, should those requirements come up. Particularly for multi-tenancy, I think this is a much more maintainable and debuggable approach.
– kungphu
Nov 13 at 5:47
Per Mr. Henry's answer, this is possible... but I would not call it advisable. It's a good idea to treat the Django admin as a low-level interface for top-level technical administration; if you have user-facing object manipulation, it's usually simpler and better to handle this with ModelForm or Form views and templates that can easily be altered/updated without worrying about what might change in the admin package, which is also a lot more complex to skin/alter, should those requirements come up. Particularly for multi-tenancy, I think this is a much more maintainable and debuggable approach.
– kungphu
Nov 13 at 5:47
add a comment |
1 Answer
1
active
oldest
votes
The documented way to do this is to define has_change_permission()
:
@admin.register(Guest)
class GuestAdmin(admin.ModelAdmin):
def get_queryset(self, request):
return super().get_queryset(request).filter(user=request.user)
def has_change_permission(self, request, obj=None):
return (obj is None or obj.user == request.user)
No need to muck about with the form.
Thank you. I was unaware of that method. Works like a champ.
– crazy4linux
Nov 13 at 12:52
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53268944%2fdoes-djangos-get-queryset-in-admin-prevent-malicious-object-saving%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The documented way to do this is to define has_change_permission()
:
@admin.register(Guest)
class GuestAdmin(admin.ModelAdmin):
def get_queryset(self, request):
return super().get_queryset(request).filter(user=request.user)
def has_change_permission(self, request, obj=None):
return (obj is None or obj.user == request.user)
No need to muck about with the form.
Thank you. I was unaware of that method. Works like a champ.
– crazy4linux
Nov 13 at 12:52
add a comment |
The documented way to do this is to define has_change_permission()
:
@admin.register(Guest)
class GuestAdmin(admin.ModelAdmin):
def get_queryset(self, request):
return super().get_queryset(request).filter(user=request.user)
def has_change_permission(self, request, obj=None):
return (obj is None or obj.user == request.user)
No need to muck about with the form.
Thank you. I was unaware of that method. Works like a champ.
– crazy4linux
Nov 13 at 12:52
add a comment |
The documented way to do this is to define has_change_permission()
:
@admin.register(Guest)
class GuestAdmin(admin.ModelAdmin):
def get_queryset(self, request):
return super().get_queryset(request).filter(user=request.user)
def has_change_permission(self, request, obj=None):
return (obj is None or obj.user == request.user)
No need to muck about with the form.
The documented way to do this is to define has_change_permission()
:
@admin.register(Guest)
class GuestAdmin(admin.ModelAdmin):
def get_queryset(self, request):
return super().get_queryset(request).filter(user=request.user)
def has_change_permission(self, request, obj=None):
return (obj is None or obj.user == request.user)
No need to muck about with the form.
answered Nov 13 at 5:42
Kevin Christopher Henry
22.5k46361
22.5k46361
Thank you. I was unaware of that method. Works like a champ.
– crazy4linux
Nov 13 at 12:52
add a comment |
Thank you. I was unaware of that method. Works like a champ.
– crazy4linux
Nov 13 at 12:52
Thank you. I was unaware of that method. Works like a champ.
– crazy4linux
Nov 13 at 12:52
Thank you. I was unaware of that method. Works like a champ.
– crazy4linux
Nov 13 at 12:52
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53268944%2fdoes-djangos-get-queryset-in-admin-prevent-malicious-object-saving%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
dmpi Hiobg kUUE2UyP,F M,iWEt
Per Mr. Henry's answer, this is possible... but I would not call it advisable. It's a good idea to treat the Django admin as a low-level interface for top-level technical administration; if you have user-facing object manipulation, it's usually simpler and better to handle this with ModelForm or Form views and templates that can easily be altered/updated without worrying about what might change in the admin package, which is also a lot more complex to skin/alter, should those requirements come up. Particularly for multi-tenancy, I think this is a much more maintainable and debuggable approach.
– kungphu
Nov 13 at 5:47