PNG loaded with SDL_image with glTexImage2D segfaults
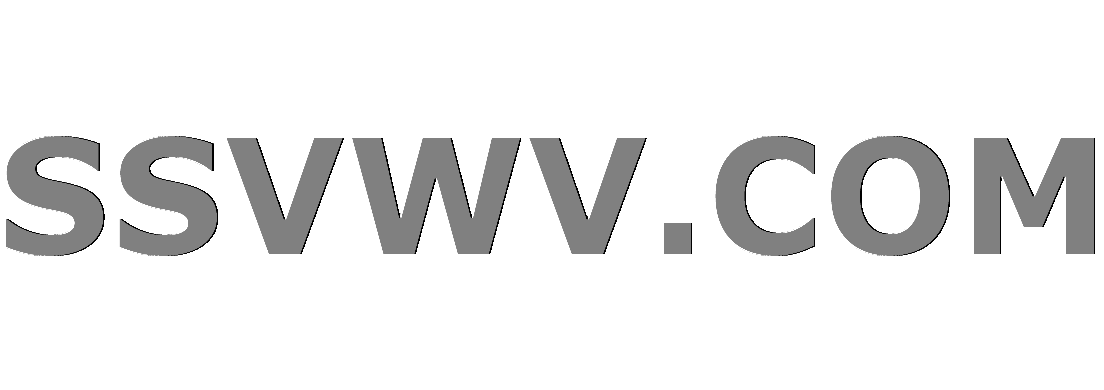
Multi tool use
up vote
1
down vote
favorite
I don't think this is a problem with the image I'm loading. The image resolution is 256x256 so it's not the power of 2 issue. I've looked at other segfaults people got with glTexImage2D and the segfault still can't seem to go away. Sorry that the code isn't in C/C++ (i don't think this is the problem either) but it should still be easy to understand.
let surface = sdlimage.load("image.png") # equivalent to IMG_Load call in C
if surface.isNil:
echo "Image couldn't be loaded: ", sdl2.getError()
quit 1
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA)
glEnable(GL_BLEND)
var tex: cuint
glGenTextures(1, addr tex)
glBindTexture(GL_TEXTURE_2D, tex)
glPixelStorei(GL_UNPACK_ALIGNMENT, 1)
glPixelStorei(GL_UNPACK_ROW_LENGTH, surface.pitch)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR)
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA.GLint, surface.w.GLsizei, surface.h.GLsizei, 0, GL_RGBA, GL_UNSIGNED_BYTE, surface.pixels) # segfault
UPDATE:
I managed to get it to not segfault thanks to user1118321's answer (I checked the pixel format and saw that SDL used a "indexed" format, which I saw another user said was the problem when they fixed this issue for theirselves. Seems like creating a new surface is the right solution), but now it shows nothing on the screen. It's black when I set glClearColor to black, and it's white when i set glClearColor to white.
Updated image loading code:
let surface = sdlimage.load("image.png")
if surface.isNil:
echo "Image couldn't be loaded: ", sdl2.getError()
quit 1
var w = surface.w # may need to make this the next power of two
var h = surface.h # and this
var bpp: cint
var Rmask, Gmask, Bmask, Amask: uint32
if not pixelFormatEnumToMasks(SDL_PIXELFORMAT_ABGR8888, bpp,
Rmask, Gmask, Bmask, Amask):
quit "pixel format enum to masks " & $sdl2.getError()
let newSurface = createRGBSurface(0, w, h, bpp,
Rmask, Gmask, Bmask, Amask)
discard surface.setSurfaceAlphaMod(0xFF)
discard surface.setSurfaceBlendMode(BlendMode_None)
blitSurface(surface, nil, newSurface, nil)
var tex: cuint
glGenTextures(1, addr tex)
glBindTexture(GL_TEXTURE_2D, tex)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR)
glTexImage2D(GL_TEXTURE_2D, 0, 4, w.GLsizei, h.GLsizei, 0, GL_RGBA, GL_UNSIGNED_BYTE, newSurface.pixels)
Image rendering code:
glUseProgram(shaderProgram)
glClear(GL_COLOR_BUFFER_BIT or GL_DEPTH_BUFFER_BIT)
glMatrixMode(GL_MODELVIEW)
glLoadIdentity()
glTranslatef(0.0, 0.0, -7.0)
glColor3f(1.0, 1.0, 1.0)
glBegin(GL_QUADS)
glTexCoord2i(0, 0)
glVertex3f(-0.5, -1.9, 0.0)
glTexCoord2i(1, 0)
glVertex3f(0.5, -1.9, 0.0)
glTexCoord2i(0, 1)
glVertex3f(-0.5, -1.4, 0.0)
glTexCoord2i(1, 1)
glVertex3f(0.5, -1.4, 0.0)
glEnd()
If shaders have something to do with it, here's my vertex shader:
#version 330 core
in vec4 data;
out vec3 ourColor;
void main() {
gl_Position = vec4(data.x, data.y, data.z, 1.0);
ourColor = vec3(data.w, data.w, data.w);
}
And the fragment shader:
#version 330 core
precision highp float;
in vec3 ourColor;
out vec4 color;
void main() {
color = vec4(ourColor, 1.0);
}
opengl sdl-2
add a comment |
up vote
1
down vote
favorite
I don't think this is a problem with the image I'm loading. The image resolution is 256x256 so it's not the power of 2 issue. I've looked at other segfaults people got with glTexImage2D and the segfault still can't seem to go away. Sorry that the code isn't in C/C++ (i don't think this is the problem either) but it should still be easy to understand.
let surface = sdlimage.load("image.png") # equivalent to IMG_Load call in C
if surface.isNil:
echo "Image couldn't be loaded: ", sdl2.getError()
quit 1
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA)
glEnable(GL_BLEND)
var tex: cuint
glGenTextures(1, addr tex)
glBindTexture(GL_TEXTURE_2D, tex)
glPixelStorei(GL_UNPACK_ALIGNMENT, 1)
glPixelStorei(GL_UNPACK_ROW_LENGTH, surface.pitch)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR)
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA.GLint, surface.w.GLsizei, surface.h.GLsizei, 0, GL_RGBA, GL_UNSIGNED_BYTE, surface.pixels) # segfault
UPDATE:
I managed to get it to not segfault thanks to user1118321's answer (I checked the pixel format and saw that SDL used a "indexed" format, which I saw another user said was the problem when they fixed this issue for theirselves. Seems like creating a new surface is the right solution), but now it shows nothing on the screen. It's black when I set glClearColor to black, and it's white when i set glClearColor to white.
Updated image loading code:
let surface = sdlimage.load("image.png")
if surface.isNil:
echo "Image couldn't be loaded: ", sdl2.getError()
quit 1
var w = surface.w # may need to make this the next power of two
var h = surface.h # and this
var bpp: cint
var Rmask, Gmask, Bmask, Amask: uint32
if not pixelFormatEnumToMasks(SDL_PIXELFORMAT_ABGR8888, bpp,
Rmask, Gmask, Bmask, Amask):
quit "pixel format enum to masks " & $sdl2.getError()
let newSurface = createRGBSurface(0, w, h, bpp,
Rmask, Gmask, Bmask, Amask)
discard surface.setSurfaceAlphaMod(0xFF)
discard surface.setSurfaceBlendMode(BlendMode_None)
blitSurface(surface, nil, newSurface, nil)
var tex: cuint
glGenTextures(1, addr tex)
glBindTexture(GL_TEXTURE_2D, tex)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR)
glTexImage2D(GL_TEXTURE_2D, 0, 4, w.GLsizei, h.GLsizei, 0, GL_RGBA, GL_UNSIGNED_BYTE, newSurface.pixels)
Image rendering code:
glUseProgram(shaderProgram)
glClear(GL_COLOR_BUFFER_BIT or GL_DEPTH_BUFFER_BIT)
glMatrixMode(GL_MODELVIEW)
glLoadIdentity()
glTranslatef(0.0, 0.0, -7.0)
glColor3f(1.0, 1.0, 1.0)
glBegin(GL_QUADS)
glTexCoord2i(0, 0)
glVertex3f(-0.5, -1.9, 0.0)
glTexCoord2i(1, 0)
glVertex3f(0.5, -1.9, 0.0)
glTexCoord2i(0, 1)
glVertex3f(-0.5, -1.4, 0.0)
glTexCoord2i(1, 1)
glVertex3f(0.5, -1.4, 0.0)
glEnd()
If shaders have something to do with it, here's my vertex shader:
#version 330 core
in vec4 data;
out vec3 ourColor;
void main() {
gl_Position = vec4(data.x, data.y, data.z, 1.0);
ourColor = vec3(data.w, data.w, data.w);
}
And the fragment shader:
#version 330 core
precision highp float;
in vec3 ourColor;
out vec4 color;
void main() {
color = vec4(ourColor, 1.0);
}
opengl sdl-2
Exactly when does the segfault occurs?
– José Manuel Ramos
Nov 10 at 21:41
In the last line.
– Hlaaftana
Nov 11 at 3:38
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I don't think this is a problem with the image I'm loading. The image resolution is 256x256 so it's not the power of 2 issue. I've looked at other segfaults people got with glTexImage2D and the segfault still can't seem to go away. Sorry that the code isn't in C/C++ (i don't think this is the problem either) but it should still be easy to understand.
let surface = sdlimage.load("image.png") # equivalent to IMG_Load call in C
if surface.isNil:
echo "Image couldn't be loaded: ", sdl2.getError()
quit 1
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA)
glEnable(GL_BLEND)
var tex: cuint
glGenTextures(1, addr tex)
glBindTexture(GL_TEXTURE_2D, tex)
glPixelStorei(GL_UNPACK_ALIGNMENT, 1)
glPixelStorei(GL_UNPACK_ROW_LENGTH, surface.pitch)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR)
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA.GLint, surface.w.GLsizei, surface.h.GLsizei, 0, GL_RGBA, GL_UNSIGNED_BYTE, surface.pixels) # segfault
UPDATE:
I managed to get it to not segfault thanks to user1118321's answer (I checked the pixel format and saw that SDL used a "indexed" format, which I saw another user said was the problem when they fixed this issue for theirselves. Seems like creating a new surface is the right solution), but now it shows nothing on the screen. It's black when I set glClearColor to black, and it's white when i set glClearColor to white.
Updated image loading code:
let surface = sdlimage.load("image.png")
if surface.isNil:
echo "Image couldn't be loaded: ", sdl2.getError()
quit 1
var w = surface.w # may need to make this the next power of two
var h = surface.h # and this
var bpp: cint
var Rmask, Gmask, Bmask, Amask: uint32
if not pixelFormatEnumToMasks(SDL_PIXELFORMAT_ABGR8888, bpp,
Rmask, Gmask, Bmask, Amask):
quit "pixel format enum to masks " & $sdl2.getError()
let newSurface = createRGBSurface(0, w, h, bpp,
Rmask, Gmask, Bmask, Amask)
discard surface.setSurfaceAlphaMod(0xFF)
discard surface.setSurfaceBlendMode(BlendMode_None)
blitSurface(surface, nil, newSurface, nil)
var tex: cuint
glGenTextures(1, addr tex)
glBindTexture(GL_TEXTURE_2D, tex)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR)
glTexImage2D(GL_TEXTURE_2D, 0, 4, w.GLsizei, h.GLsizei, 0, GL_RGBA, GL_UNSIGNED_BYTE, newSurface.pixels)
Image rendering code:
glUseProgram(shaderProgram)
glClear(GL_COLOR_BUFFER_BIT or GL_DEPTH_BUFFER_BIT)
glMatrixMode(GL_MODELVIEW)
glLoadIdentity()
glTranslatef(0.0, 0.0, -7.0)
glColor3f(1.0, 1.0, 1.0)
glBegin(GL_QUADS)
glTexCoord2i(0, 0)
glVertex3f(-0.5, -1.9, 0.0)
glTexCoord2i(1, 0)
glVertex3f(0.5, -1.9, 0.0)
glTexCoord2i(0, 1)
glVertex3f(-0.5, -1.4, 0.0)
glTexCoord2i(1, 1)
glVertex3f(0.5, -1.4, 0.0)
glEnd()
If shaders have something to do with it, here's my vertex shader:
#version 330 core
in vec4 data;
out vec3 ourColor;
void main() {
gl_Position = vec4(data.x, data.y, data.z, 1.0);
ourColor = vec3(data.w, data.w, data.w);
}
And the fragment shader:
#version 330 core
precision highp float;
in vec3 ourColor;
out vec4 color;
void main() {
color = vec4(ourColor, 1.0);
}
opengl sdl-2
I don't think this is a problem with the image I'm loading. The image resolution is 256x256 so it's not the power of 2 issue. I've looked at other segfaults people got with glTexImage2D and the segfault still can't seem to go away. Sorry that the code isn't in C/C++ (i don't think this is the problem either) but it should still be easy to understand.
let surface = sdlimage.load("image.png") # equivalent to IMG_Load call in C
if surface.isNil:
echo "Image couldn't be loaded: ", sdl2.getError()
quit 1
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA)
glEnable(GL_BLEND)
var tex: cuint
glGenTextures(1, addr tex)
glBindTexture(GL_TEXTURE_2D, tex)
glPixelStorei(GL_UNPACK_ALIGNMENT, 1)
glPixelStorei(GL_UNPACK_ROW_LENGTH, surface.pitch)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR)
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA.GLint, surface.w.GLsizei, surface.h.GLsizei, 0, GL_RGBA, GL_UNSIGNED_BYTE, surface.pixels) # segfault
UPDATE:
I managed to get it to not segfault thanks to user1118321's answer (I checked the pixel format and saw that SDL used a "indexed" format, which I saw another user said was the problem when they fixed this issue for theirselves. Seems like creating a new surface is the right solution), but now it shows nothing on the screen. It's black when I set glClearColor to black, and it's white when i set glClearColor to white.
Updated image loading code:
let surface = sdlimage.load("image.png")
if surface.isNil:
echo "Image couldn't be loaded: ", sdl2.getError()
quit 1
var w = surface.w # may need to make this the next power of two
var h = surface.h # and this
var bpp: cint
var Rmask, Gmask, Bmask, Amask: uint32
if not pixelFormatEnumToMasks(SDL_PIXELFORMAT_ABGR8888, bpp,
Rmask, Gmask, Bmask, Amask):
quit "pixel format enum to masks " & $sdl2.getError()
let newSurface = createRGBSurface(0, w, h, bpp,
Rmask, Gmask, Bmask, Amask)
discard surface.setSurfaceAlphaMod(0xFF)
discard surface.setSurfaceBlendMode(BlendMode_None)
blitSurface(surface, nil, newSurface, nil)
var tex: cuint
glGenTextures(1, addr tex)
glBindTexture(GL_TEXTURE_2D, tex)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR)
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR)
glTexImage2D(GL_TEXTURE_2D, 0, 4, w.GLsizei, h.GLsizei, 0, GL_RGBA, GL_UNSIGNED_BYTE, newSurface.pixels)
Image rendering code:
glUseProgram(shaderProgram)
glClear(GL_COLOR_BUFFER_BIT or GL_DEPTH_BUFFER_BIT)
glMatrixMode(GL_MODELVIEW)
glLoadIdentity()
glTranslatef(0.0, 0.0, -7.0)
glColor3f(1.0, 1.0, 1.0)
glBegin(GL_QUADS)
glTexCoord2i(0, 0)
glVertex3f(-0.5, -1.9, 0.0)
glTexCoord2i(1, 0)
glVertex3f(0.5, -1.9, 0.0)
glTexCoord2i(0, 1)
glVertex3f(-0.5, -1.4, 0.0)
glTexCoord2i(1, 1)
glVertex3f(0.5, -1.4, 0.0)
glEnd()
If shaders have something to do with it, here's my vertex shader:
#version 330 core
in vec4 data;
out vec3 ourColor;
void main() {
gl_Position = vec4(data.x, data.y, data.z, 1.0);
ourColor = vec3(data.w, data.w, data.w);
}
And the fragment shader:
#version 330 core
precision highp float;
in vec3 ourColor;
out vec4 color;
void main() {
color = vec4(ourColor, 1.0);
}
opengl sdl-2
opengl sdl-2
edited Nov 11 at 4:27
asked Nov 10 at 20:42
Hlaaftana
62
62
Exactly when does the segfault occurs?
– José Manuel Ramos
Nov 10 at 21:41
In the last line.
– Hlaaftana
Nov 11 at 3:38
add a comment |
Exactly when does the segfault occurs?
– José Manuel Ramos
Nov 10 at 21:41
In the last line.
– Hlaaftana
Nov 11 at 3:38
Exactly when does the segfault occurs?
– José Manuel Ramos
Nov 10 at 21:41
Exactly when does the segfault occurs?
– José Manuel Ramos
Nov 10 at 21:41
In the last line.
– Hlaaftana
Nov 11 at 3:38
In the last line.
– Hlaaftana
Nov 11 at 3:38
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
You should check glGetError()
to see if there are any OpenGL errors occurring. You should also check the surface.pixelFormat
to see whether you actually have 4 bytes per pixel in your image. If it's just RGB data and not RGBA, then glTexImage2D()
will read past the end of the image data and likely crash with a segmentation fault.
Thank you for the tip, I managed to get it to not segfault, but now the image doesn't seem to render. I've put code in the update in my post.
– Hlaaftana
Nov 11 at 4:28
2
That's because you aren't actually sampling the image. You're just outputting a solid color in your fragment shader.
– user1118321
Nov 11 at 4:46
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
You should check glGetError()
to see if there are any OpenGL errors occurring. You should also check the surface.pixelFormat
to see whether you actually have 4 bytes per pixel in your image. If it's just RGB data and not RGBA, then glTexImage2D()
will read past the end of the image data and likely crash with a segmentation fault.
Thank you for the tip, I managed to get it to not segfault, but now the image doesn't seem to render. I've put code in the update in my post.
– Hlaaftana
Nov 11 at 4:28
2
That's because you aren't actually sampling the image. You're just outputting a solid color in your fragment shader.
– user1118321
Nov 11 at 4:46
add a comment |
up vote
1
down vote
You should check glGetError()
to see if there are any OpenGL errors occurring. You should also check the surface.pixelFormat
to see whether you actually have 4 bytes per pixel in your image. If it's just RGB data and not RGBA, then glTexImage2D()
will read past the end of the image data and likely crash with a segmentation fault.
Thank you for the tip, I managed to get it to not segfault, but now the image doesn't seem to render. I've put code in the update in my post.
– Hlaaftana
Nov 11 at 4:28
2
That's because you aren't actually sampling the image. You're just outputting a solid color in your fragment shader.
– user1118321
Nov 11 at 4:46
add a comment |
up vote
1
down vote
up vote
1
down vote
You should check glGetError()
to see if there are any OpenGL errors occurring. You should also check the surface.pixelFormat
to see whether you actually have 4 bytes per pixel in your image. If it's just RGB data and not RGBA, then glTexImage2D()
will read past the end of the image data and likely crash with a segmentation fault.
You should check glGetError()
to see if there are any OpenGL errors occurring. You should also check the surface.pixelFormat
to see whether you actually have 4 bytes per pixel in your image. If it's just RGB data and not RGBA, then glTexImage2D()
will read past the end of the image data and likely crash with a segmentation fault.
answered Nov 10 at 21:41
user1118321
19.1k43862
19.1k43862
Thank you for the tip, I managed to get it to not segfault, but now the image doesn't seem to render. I've put code in the update in my post.
– Hlaaftana
Nov 11 at 4:28
2
That's because you aren't actually sampling the image. You're just outputting a solid color in your fragment shader.
– user1118321
Nov 11 at 4:46
add a comment |
Thank you for the tip, I managed to get it to not segfault, but now the image doesn't seem to render. I've put code in the update in my post.
– Hlaaftana
Nov 11 at 4:28
2
That's because you aren't actually sampling the image. You're just outputting a solid color in your fragment shader.
– user1118321
Nov 11 at 4:46
Thank you for the tip, I managed to get it to not segfault, but now the image doesn't seem to render. I've put code in the update in my post.
– Hlaaftana
Nov 11 at 4:28
Thank you for the tip, I managed to get it to not segfault, but now the image doesn't seem to render. I've put code in the update in my post.
– Hlaaftana
Nov 11 at 4:28
2
2
That's because you aren't actually sampling the image. You're just outputting a solid color in your fragment shader.
– user1118321
Nov 11 at 4:46
That's because you aren't actually sampling the image. You're just outputting a solid color in your fragment shader.
– user1118321
Nov 11 at 4:46
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53243221%2fpng-loaded-with-sdl-image-with-glteximage2d-segfaults%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eQkWorLKu0MG1QLm5Dnt9 0 wXiWTydr
Exactly when does the segfault occurs?
– José Manuel Ramos
Nov 10 at 21:41
In the last line.
– Hlaaftana
Nov 11 at 3:38