Adding Custom Search Fields to a Datatable Object
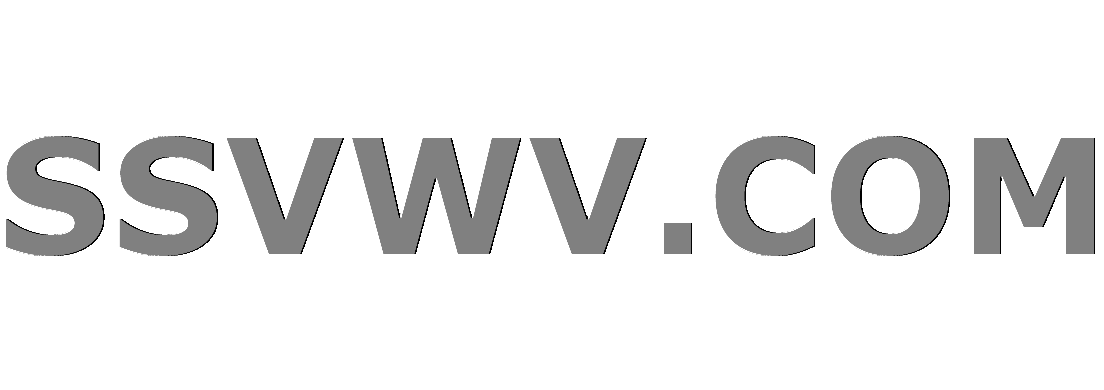
Multi tool use
up vote
5
down vote
favorite
I need to add fields that I created on a page to the Datatables object. So that even though they aren't typical parameters (order, search, pagination, etc.) they can get save and loaded along with the rest of the Datatables object state.
JavaScript:
$(document).ready(function () {
var table = $('#stackoverflow-datatable').DataTable({
"processing": true,
"stateSave": true,
"stateSaveCallback": function (settings, data) {
$.ajax({
"url": "/api/save_state",
"data": data,
"dataType": "json",
"success": function (response) {}
});
},
"stateLoadCallback": function (settings) {
var o;
$.ajax({
"url": "/api/load_state",
"async": false,
"dataType": "json",
"success": function (json) {
o = json;
}
});
return o;
}
});
});
And then the JS that I thought would add the fields to the object...
<script type="text/javascript">
$.fn.dataTableExt.afnFiltering.push(
function (settings, data) {
var dateStart = parseDateValue($("#dateStart").val());
var dateEnd = parseDateValue($("#dateEnd").val());
var evalDate = parseDateValue(data[9]);
return evalDate >= dateStart && evalDate <= dateEnd;
}
);
function getDate(element) {
return $.datepicker.parseDate('mm/dd/yy', element.value);
}
// Convert mm/dd/yyyy into numeric (ex. 08/12/2010 -> 20100812)
function parseDateValue(rawDate) {
var dateArray = rawDate.split("/");
return dateArray[2] + dateArray[0] + dateArray[1];
}
</script>
HTML:
<input type="text" id="dateStart" class="datepicker" name="dateStart">
<input type="text" id="dateEnd" class="datepicker" name="dateEnd">
<table id="stackoverflow-datatable">
<thead>
<th>ID</th>
<th>Name</th>
<th>Date</th>
</thead>
...
Thanks in advance for your help.
javascript jquery datatables
add a comment |
up vote
5
down vote
favorite
I need to add fields that I created on a page to the Datatables object. So that even though they aren't typical parameters (order, search, pagination, etc.) they can get save and loaded along with the rest of the Datatables object state.
JavaScript:
$(document).ready(function () {
var table = $('#stackoverflow-datatable').DataTable({
"processing": true,
"stateSave": true,
"stateSaveCallback": function (settings, data) {
$.ajax({
"url": "/api/save_state",
"data": data,
"dataType": "json",
"success": function (response) {}
});
},
"stateLoadCallback": function (settings) {
var o;
$.ajax({
"url": "/api/load_state",
"async": false,
"dataType": "json",
"success": function (json) {
o = json;
}
});
return o;
}
});
});
And then the JS that I thought would add the fields to the object...
<script type="text/javascript">
$.fn.dataTableExt.afnFiltering.push(
function (settings, data) {
var dateStart = parseDateValue($("#dateStart").val());
var dateEnd = parseDateValue($("#dateEnd").val());
var evalDate = parseDateValue(data[9]);
return evalDate >= dateStart && evalDate <= dateEnd;
}
);
function getDate(element) {
return $.datepicker.parseDate('mm/dd/yy', element.value);
}
// Convert mm/dd/yyyy into numeric (ex. 08/12/2010 -> 20100812)
function parseDateValue(rawDate) {
var dateArray = rawDate.split("/");
return dateArray[2] + dateArray[0] + dateArray[1];
}
</script>
HTML:
<input type="text" id="dateStart" class="datepicker" name="dateStart">
<input type="text" id="dateEnd" class="datepicker" name="dateEnd">
<table id="stackoverflow-datatable">
<thead>
<th>ID</th>
<th>Name</th>
<th>Date</th>
</thead>
...
Thanks in advance for your help.
javascript jquery datatables
add a comment |
up vote
5
down vote
favorite
up vote
5
down vote
favorite
I need to add fields that I created on a page to the Datatables object. So that even though they aren't typical parameters (order, search, pagination, etc.) they can get save and loaded along with the rest of the Datatables object state.
JavaScript:
$(document).ready(function () {
var table = $('#stackoverflow-datatable').DataTable({
"processing": true,
"stateSave": true,
"stateSaveCallback": function (settings, data) {
$.ajax({
"url": "/api/save_state",
"data": data,
"dataType": "json",
"success": function (response) {}
});
},
"stateLoadCallback": function (settings) {
var o;
$.ajax({
"url": "/api/load_state",
"async": false,
"dataType": "json",
"success": function (json) {
o = json;
}
});
return o;
}
});
});
And then the JS that I thought would add the fields to the object...
<script type="text/javascript">
$.fn.dataTableExt.afnFiltering.push(
function (settings, data) {
var dateStart = parseDateValue($("#dateStart").val());
var dateEnd = parseDateValue($("#dateEnd").val());
var evalDate = parseDateValue(data[9]);
return evalDate >= dateStart && evalDate <= dateEnd;
}
);
function getDate(element) {
return $.datepicker.parseDate('mm/dd/yy', element.value);
}
// Convert mm/dd/yyyy into numeric (ex. 08/12/2010 -> 20100812)
function parseDateValue(rawDate) {
var dateArray = rawDate.split("/");
return dateArray[2] + dateArray[0] + dateArray[1];
}
</script>
HTML:
<input type="text" id="dateStart" class="datepicker" name="dateStart">
<input type="text" id="dateEnd" class="datepicker" name="dateEnd">
<table id="stackoverflow-datatable">
<thead>
<th>ID</th>
<th>Name</th>
<th>Date</th>
</thead>
...
Thanks in advance for your help.
javascript jquery datatables
I need to add fields that I created on a page to the Datatables object. So that even though they aren't typical parameters (order, search, pagination, etc.) they can get save and loaded along with the rest of the Datatables object state.
JavaScript:
$(document).ready(function () {
var table = $('#stackoverflow-datatable').DataTable({
"processing": true,
"stateSave": true,
"stateSaveCallback": function (settings, data) {
$.ajax({
"url": "/api/save_state",
"data": data,
"dataType": "json",
"success": function (response) {}
});
},
"stateLoadCallback": function (settings) {
var o;
$.ajax({
"url": "/api/load_state",
"async": false,
"dataType": "json",
"success": function (json) {
o = json;
}
});
return o;
}
});
});
And then the JS that I thought would add the fields to the object...
<script type="text/javascript">
$.fn.dataTableExt.afnFiltering.push(
function (settings, data) {
var dateStart = parseDateValue($("#dateStart").val());
var dateEnd = parseDateValue($("#dateEnd").val());
var evalDate = parseDateValue(data[9]);
return evalDate >= dateStart && evalDate <= dateEnd;
}
);
function getDate(element) {
return $.datepicker.parseDate('mm/dd/yy', element.value);
}
// Convert mm/dd/yyyy into numeric (ex. 08/12/2010 -> 20100812)
function parseDateValue(rawDate) {
var dateArray = rawDate.split("/");
return dateArray[2] + dateArray[0] + dateArray[1];
}
</script>
HTML:
<input type="text" id="dateStart" class="datepicker" name="dateStart">
<input type="text" id="dateEnd" class="datepicker" name="dateEnd">
<table id="stackoverflow-datatable">
<thead>
<th>ID</th>
<th>Name</th>
<th>Date</th>
</thead>
...
Thanks in advance for your help.
javascript jquery datatables
javascript jquery datatables
edited Nov 10 at 20:30
asked Dec 8 '16 at 13:37


Karl Hill
1,7491736
1,7491736
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
To implement a custom range search, use:
$.fn.dataTable.ext.search.push
To save and load custom state parameters, use:
stateSaveParams: function (settings, data) {}
stateLoadParams: function (settings, data) {}
You must attach event listeners to your datepickers, so that the table is redrawn every time the start or ending dates are changed, but you must also fill in the input fields when the table state is restored. This can be tricky because there's a cross-dependency between the pickers and the table, so I've introduced a custom event to make sure the datepickers state is restored after their creation.
I've created a working example based on your code, using a static table:
JSFIDDLE
So 'search.push' adds these to the DataTable object? Have you tried going to a different page and coming back to see if it remembers values? Because everything you have in your JSFiddle I'm using and it works perfect for filtering/searching. However, the DataTables search field remembers its values but the added custom fields: startDate, endDate are still not getting added to the "search" area in the Datable output object.
– Karl Hill
Dec 9 '16 at 21:40
1
No, you need additional API methods for that. I've updated my answer and my code to include all your requirements. I've tested the script on localhost and the table state, along with the custom fields are preserved.
– R.Costa
Dec 10 '16 at 3:46
This is a perfect answer, thank you.
– Karl Hill
Dec 10 '16 at 21:29
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
To implement a custom range search, use:
$.fn.dataTable.ext.search.push
To save and load custom state parameters, use:
stateSaveParams: function (settings, data) {}
stateLoadParams: function (settings, data) {}
You must attach event listeners to your datepickers, so that the table is redrawn every time the start or ending dates are changed, but you must also fill in the input fields when the table state is restored. This can be tricky because there's a cross-dependency between the pickers and the table, so I've introduced a custom event to make sure the datepickers state is restored after their creation.
I've created a working example based on your code, using a static table:
JSFIDDLE
So 'search.push' adds these to the DataTable object? Have you tried going to a different page and coming back to see if it remembers values? Because everything you have in your JSFiddle I'm using and it works perfect for filtering/searching. However, the DataTables search field remembers its values but the added custom fields: startDate, endDate are still not getting added to the "search" area in the Datable output object.
– Karl Hill
Dec 9 '16 at 21:40
1
No, you need additional API methods for that. I've updated my answer and my code to include all your requirements. I've tested the script on localhost and the table state, along with the custom fields are preserved.
– R.Costa
Dec 10 '16 at 3:46
This is a perfect answer, thank you.
– Karl Hill
Dec 10 '16 at 21:29
add a comment |
up vote
2
down vote
accepted
To implement a custom range search, use:
$.fn.dataTable.ext.search.push
To save and load custom state parameters, use:
stateSaveParams: function (settings, data) {}
stateLoadParams: function (settings, data) {}
You must attach event listeners to your datepickers, so that the table is redrawn every time the start or ending dates are changed, but you must also fill in the input fields when the table state is restored. This can be tricky because there's a cross-dependency between the pickers and the table, so I've introduced a custom event to make sure the datepickers state is restored after their creation.
I've created a working example based on your code, using a static table:
JSFIDDLE
So 'search.push' adds these to the DataTable object? Have you tried going to a different page and coming back to see if it remembers values? Because everything you have in your JSFiddle I'm using and it works perfect for filtering/searching. However, the DataTables search field remembers its values but the added custom fields: startDate, endDate are still not getting added to the "search" area in the Datable output object.
– Karl Hill
Dec 9 '16 at 21:40
1
No, you need additional API methods for that. I've updated my answer and my code to include all your requirements. I've tested the script on localhost and the table state, along with the custom fields are preserved.
– R.Costa
Dec 10 '16 at 3:46
This is a perfect answer, thank you.
– Karl Hill
Dec 10 '16 at 21:29
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
To implement a custom range search, use:
$.fn.dataTable.ext.search.push
To save and load custom state parameters, use:
stateSaveParams: function (settings, data) {}
stateLoadParams: function (settings, data) {}
You must attach event listeners to your datepickers, so that the table is redrawn every time the start or ending dates are changed, but you must also fill in the input fields when the table state is restored. This can be tricky because there's a cross-dependency between the pickers and the table, so I've introduced a custom event to make sure the datepickers state is restored after their creation.
I've created a working example based on your code, using a static table:
JSFIDDLE
To implement a custom range search, use:
$.fn.dataTable.ext.search.push
To save and load custom state parameters, use:
stateSaveParams: function (settings, data) {}
stateLoadParams: function (settings, data) {}
You must attach event listeners to your datepickers, so that the table is redrawn every time the start or ending dates are changed, but you must also fill in the input fields when the table state is restored. This can be tricky because there's a cross-dependency between the pickers and the table, so I've introduced a custom event to make sure the datepickers state is restored after their creation.
I've created a working example based on your code, using a static table:
JSFIDDLE
edited Dec 10 '16 at 3:26
answered Dec 9 '16 at 15:30
R.Costa
1,235515
1,235515
So 'search.push' adds these to the DataTable object? Have you tried going to a different page and coming back to see if it remembers values? Because everything you have in your JSFiddle I'm using and it works perfect for filtering/searching. However, the DataTables search field remembers its values but the added custom fields: startDate, endDate are still not getting added to the "search" area in the Datable output object.
– Karl Hill
Dec 9 '16 at 21:40
1
No, you need additional API methods for that. I've updated my answer and my code to include all your requirements. I've tested the script on localhost and the table state, along with the custom fields are preserved.
– R.Costa
Dec 10 '16 at 3:46
This is a perfect answer, thank you.
– Karl Hill
Dec 10 '16 at 21:29
add a comment |
So 'search.push' adds these to the DataTable object? Have you tried going to a different page and coming back to see if it remembers values? Because everything you have in your JSFiddle I'm using and it works perfect for filtering/searching. However, the DataTables search field remembers its values but the added custom fields: startDate, endDate are still not getting added to the "search" area in the Datable output object.
– Karl Hill
Dec 9 '16 at 21:40
1
No, you need additional API methods for that. I've updated my answer and my code to include all your requirements. I've tested the script on localhost and the table state, along with the custom fields are preserved.
– R.Costa
Dec 10 '16 at 3:46
This is a perfect answer, thank you.
– Karl Hill
Dec 10 '16 at 21:29
So 'search.push' adds these to the DataTable object? Have you tried going to a different page and coming back to see if it remembers values? Because everything you have in your JSFiddle I'm using and it works perfect for filtering/searching. However, the DataTables search field remembers its values but the added custom fields: startDate, endDate are still not getting added to the "search" area in the Datable output object.
– Karl Hill
Dec 9 '16 at 21:40
So 'search.push' adds these to the DataTable object? Have you tried going to a different page and coming back to see if it remembers values? Because everything you have in your JSFiddle I'm using and it works perfect for filtering/searching. However, the DataTables search field remembers its values but the added custom fields: startDate, endDate are still not getting added to the "search" area in the Datable output object.
– Karl Hill
Dec 9 '16 at 21:40
1
1
No, you need additional API methods for that. I've updated my answer and my code to include all your requirements. I've tested the script on localhost and the table state, along with the custom fields are preserved.
– R.Costa
Dec 10 '16 at 3:46
No, you need additional API methods for that. I've updated my answer and my code to include all your requirements. I've tested the script on localhost and the table state, along with the custom fields are preserved.
– R.Costa
Dec 10 '16 at 3:46
This is a perfect answer, thank you.
– Karl Hill
Dec 10 '16 at 21:29
This is a perfect answer, thank you.
– Karl Hill
Dec 10 '16 at 21:29
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f41040855%2fadding-custom-search-fields-to-a-datatable-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
iLjuQ4xp,F