Result order in Spring Data Elasticsearch @Query
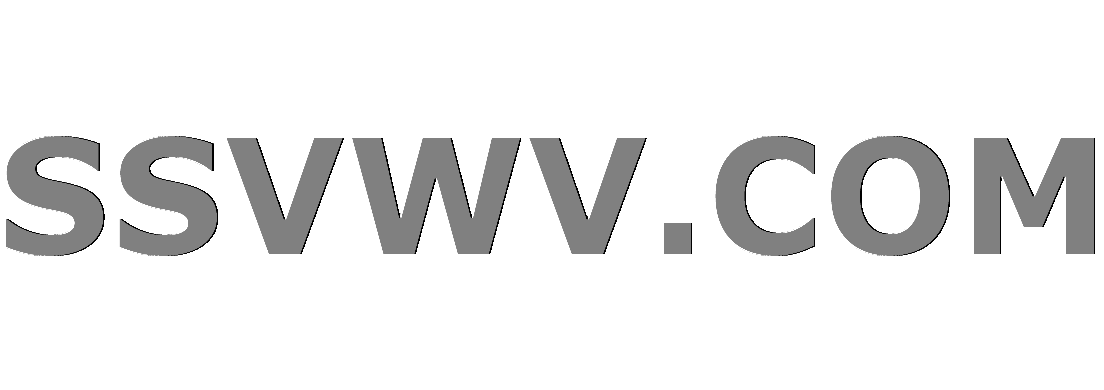
Multi tool use
This is Spring Boot Data Elasticsearch (Embedded Elasticsearch).
I have a simple query:
{
"query": {
"match_phrase" : {
"text" : "12"
}
}
}
And when I send this query via localhost:9200 there's an answer:
{
"took": 56, "timed_out": false,
"_shards": {"total": 1, "successful": 1, "failed": 0 },
"hits": {
"total": 5,
"max_score": 0.3172162,
"hits": [
{
"_index": "abc", "_type": "abc", "_id": "AWbzvDOghegm62vg48M5",
"_score": 0.3172162,
"_source": {
"entityId": "5840", "text": "abc"
}
},
{
"_score": 0.3172162,
"_source": {"entityId": "5838"}
},
{
"_score": 0.3172162,
"_source": {"entityId": "5834"}
},
{
"_score": 0.26434684,
"_source": {"entityId": "5850"}
},
{
"_score": 0.26434684,
"_source": {"entityId": "5846"}
}
]
}
}
Everything is OK.
In project I have a Data ElasticsearchRepository:
public interface MyESEntityRepository extends ElasticsearchRepository<MyESEntity, String> {
@Query("same ES query")
List<MyESEntity> find(String q);
}
And here my problem: I sand the same query and receive same set but in a different order.
Through 9200 I receive next ordered by _score
set with entityId
: 5840
5838
5834
5850
5846
.
ES Data Repository return: 5850
5846
5840
5838
5834
. Thus, in this example, it looks as if it is a desc order by entityId. However, in a real query, something is different. Perhaps this is the order of time modification.
How to get order by _score
?
With
Pageable
same effect:Page<MyESEntity> find(String q, Pageable pageable);
and
find("12", new PageRequest(0, 10, new Sort(new Sort.Order(Sort.Direction.DESC,"_score")))).getContent();
java spring-data-elasticsearch
add a comment |
This is Spring Boot Data Elasticsearch (Embedded Elasticsearch).
I have a simple query:
{
"query": {
"match_phrase" : {
"text" : "12"
}
}
}
And when I send this query via localhost:9200 there's an answer:
{
"took": 56, "timed_out": false,
"_shards": {"total": 1, "successful": 1, "failed": 0 },
"hits": {
"total": 5,
"max_score": 0.3172162,
"hits": [
{
"_index": "abc", "_type": "abc", "_id": "AWbzvDOghegm62vg48M5",
"_score": 0.3172162,
"_source": {
"entityId": "5840", "text": "abc"
}
},
{
"_score": 0.3172162,
"_source": {"entityId": "5838"}
},
{
"_score": 0.3172162,
"_source": {"entityId": "5834"}
},
{
"_score": 0.26434684,
"_source": {"entityId": "5850"}
},
{
"_score": 0.26434684,
"_source": {"entityId": "5846"}
}
]
}
}
Everything is OK.
In project I have a Data ElasticsearchRepository:
public interface MyESEntityRepository extends ElasticsearchRepository<MyESEntity, String> {
@Query("same ES query")
List<MyESEntity> find(String q);
}
And here my problem: I sand the same query and receive same set but in a different order.
Through 9200 I receive next ordered by _score
set with entityId
: 5840
5838
5834
5850
5846
.
ES Data Repository return: 5850
5846
5840
5838
5834
. Thus, in this example, it looks as if it is a desc order by entityId. However, in a real query, something is different. Perhaps this is the order of time modification.
How to get order by _score
?
With
Pageable
same effect:Page<MyESEntity> find(String q, Pageable pageable);
and
find("12", new PageRequest(0, 10, new Sort(new Sort.Order(Sort.Direction.DESC,"_score")))).getContent();
java spring-data-elasticsearch
I think already answered here: stackoverflow.com/questions/28797075/…
– jprogramista
Nov 15 '18 at 12:00
@jprogramista There is an example of sorting by the fields of the entity. Immediately there is a temporary _score field is not sure that it can be accessed. But thanks, I'll try.
– Shklyar
Nov 15 '18 at 12:27
add a comment |
This is Spring Boot Data Elasticsearch (Embedded Elasticsearch).
I have a simple query:
{
"query": {
"match_phrase" : {
"text" : "12"
}
}
}
And when I send this query via localhost:9200 there's an answer:
{
"took": 56, "timed_out": false,
"_shards": {"total": 1, "successful": 1, "failed": 0 },
"hits": {
"total": 5,
"max_score": 0.3172162,
"hits": [
{
"_index": "abc", "_type": "abc", "_id": "AWbzvDOghegm62vg48M5",
"_score": 0.3172162,
"_source": {
"entityId": "5840", "text": "abc"
}
},
{
"_score": 0.3172162,
"_source": {"entityId": "5838"}
},
{
"_score": 0.3172162,
"_source": {"entityId": "5834"}
},
{
"_score": 0.26434684,
"_source": {"entityId": "5850"}
},
{
"_score": 0.26434684,
"_source": {"entityId": "5846"}
}
]
}
}
Everything is OK.
In project I have a Data ElasticsearchRepository:
public interface MyESEntityRepository extends ElasticsearchRepository<MyESEntity, String> {
@Query("same ES query")
List<MyESEntity> find(String q);
}
And here my problem: I sand the same query and receive same set but in a different order.
Through 9200 I receive next ordered by _score
set with entityId
: 5840
5838
5834
5850
5846
.
ES Data Repository return: 5850
5846
5840
5838
5834
. Thus, in this example, it looks as if it is a desc order by entityId. However, in a real query, something is different. Perhaps this is the order of time modification.
How to get order by _score
?
With
Pageable
same effect:Page<MyESEntity> find(String q, Pageable pageable);
and
find("12", new PageRequest(0, 10, new Sort(new Sort.Order(Sort.Direction.DESC,"_score")))).getContent();
java spring-data-elasticsearch
This is Spring Boot Data Elasticsearch (Embedded Elasticsearch).
I have a simple query:
{
"query": {
"match_phrase" : {
"text" : "12"
}
}
}
And when I send this query via localhost:9200 there's an answer:
{
"took": 56, "timed_out": false,
"_shards": {"total": 1, "successful": 1, "failed": 0 },
"hits": {
"total": 5,
"max_score": 0.3172162,
"hits": [
{
"_index": "abc", "_type": "abc", "_id": "AWbzvDOghegm62vg48M5",
"_score": 0.3172162,
"_source": {
"entityId": "5840", "text": "abc"
}
},
{
"_score": 0.3172162,
"_source": {"entityId": "5838"}
},
{
"_score": 0.3172162,
"_source": {"entityId": "5834"}
},
{
"_score": 0.26434684,
"_source": {"entityId": "5850"}
},
{
"_score": 0.26434684,
"_source": {"entityId": "5846"}
}
]
}
}
Everything is OK.
In project I have a Data ElasticsearchRepository:
public interface MyESEntityRepository extends ElasticsearchRepository<MyESEntity, String> {
@Query("same ES query")
List<MyESEntity> find(String q);
}
And here my problem: I sand the same query and receive same set but in a different order.
Through 9200 I receive next ordered by _score
set with entityId
: 5840
5838
5834
5850
5846
.
ES Data Repository return: 5850
5846
5840
5838
5834
. Thus, in this example, it looks as if it is a desc order by entityId. However, in a real query, something is different. Perhaps this is the order of time modification.
How to get order by _score
?
With
Pageable
same effect:Page<MyESEntity> find(String q, Pageable pageable);
and
find("12", new PageRequest(0, 10, new Sort(new Sort.Order(Sort.Direction.DESC,"_score")))).getContent();
java spring-data-elasticsearch
java spring-data-elasticsearch
edited Nov 15 '18 at 13:26
Shklyar
asked Nov 15 '18 at 11:49


ShklyarShklyar
4610
4610
I think already answered here: stackoverflow.com/questions/28797075/…
– jprogramista
Nov 15 '18 at 12:00
@jprogramista There is an example of sorting by the fields of the entity. Immediately there is a temporary _score field is not sure that it can be accessed. But thanks, I'll try.
– Shklyar
Nov 15 '18 at 12:27
add a comment |
I think already answered here: stackoverflow.com/questions/28797075/…
– jprogramista
Nov 15 '18 at 12:00
@jprogramista There is an example of sorting by the fields of the entity. Immediately there is a temporary _score field is not sure that it can be accessed. But thanks, I'll try.
– Shklyar
Nov 15 '18 at 12:27
I think already answered here: stackoverflow.com/questions/28797075/…
– jprogramista
Nov 15 '18 at 12:00
I think already answered here: stackoverflow.com/questions/28797075/…
– jprogramista
Nov 15 '18 at 12:00
@jprogramista There is an example of sorting by the fields of the entity. Immediately there is a temporary _score field is not sure that it can be accessed. But thanks, I'll try.
– Shklyar
Nov 15 '18 at 12:27
@jprogramista There is an example of sorting by the fields of the entity. Immediately there is a temporary _score field is not sure that it can be accessed. But thanks, I'll try.
– Shklyar
Nov 15 '18 at 12:27
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53318831%2fresult-order-in-spring-data-elasticsearch-query%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53318831%2fresult-order-in-spring-data-elasticsearch-query%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5bRMHXpDu9yYAD3iOeyHu0b9r6 c7Z3 7g4lBipfW5mQEXHeRKAxAhb
I think already answered here: stackoverflow.com/questions/28797075/…
– jprogramista
Nov 15 '18 at 12:00
@jprogramista There is an example of sorting by the fields of the entity. Immediately there is a temporary _score field is not sure that it can be accessed. But thanks, I'll try.
– Shklyar
Nov 15 '18 at 12:27