How to create a .json file from fetch API call response using fs.writeFile
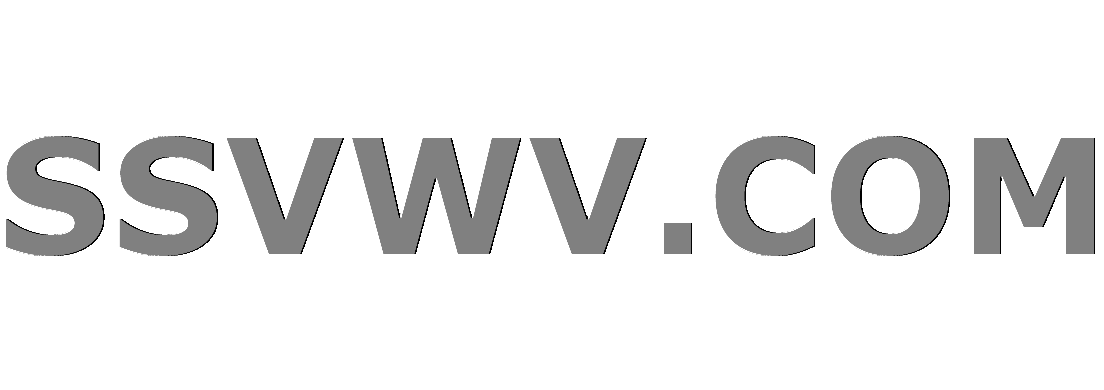
Multi tool use
I want to generate a .json file on my local project folder. I want to save the fetch API call response (which is an array of objects) into a .json file on my hard disc. Here is my code :
const fs = require('fs');
export default function getdirectory(token) {
return fetch(url, {
headers: {Authorization: "Bearer " + token}
})
.then((response) => response.json())
.catch((err) =>{
console.log("ERORRRRRRRR @@@@@@" + err);
})
.then((response) => {
//console.log(response);
var jsoncontent = JSON.stringify(response);
//console.log(jsoncontent);
fs.writeFileSync('files-to-cache.json', jsoncontent, function(err) {
if (err)
{console.log("EROROROROROR ****" + err);}
else
{ return response;}
});
// return response;
})
.catch((err) =>{
console.log("ERORRRRRRRR $$$$$$" + err);
})
};
When I run the code in vs code I get the following error:
undefined is not a function (near '...fs.writeFileSync...')
It seems that it happens because the data is not ready at the time of json file creation (asynchronous). Some web sites recommend setTimeOut function or sf.writeFileSync instead of sf.writeFile. I tried both, but still it doesn't work.
Would you please help me to resolve this problem?
Many thanks
javascript
add a comment |
I want to generate a .json file on my local project folder. I want to save the fetch API call response (which is an array of objects) into a .json file on my hard disc. Here is my code :
const fs = require('fs');
export default function getdirectory(token) {
return fetch(url, {
headers: {Authorization: "Bearer " + token}
})
.then((response) => response.json())
.catch((err) =>{
console.log("ERORRRRRRRR @@@@@@" + err);
})
.then((response) => {
//console.log(response);
var jsoncontent = JSON.stringify(response);
//console.log(jsoncontent);
fs.writeFileSync('files-to-cache.json', jsoncontent, function(err) {
if (err)
{console.log("EROROROROROR ****" + err);}
else
{ return response;}
});
// return response;
})
.catch((err) =>{
console.log("ERORRRRRRRR $$$$$$" + err);
})
};
When I run the code in vs code I get the following error:
undefined is not a function (near '...fs.writeFileSync...')
It seems that it happens because the data is not ready at the time of json file creation (asynchronous). Some web sites recommend setTimeOut function or sf.writeFileSync instead of sf.writeFile. I tried both, but still it doesn't work.
Would you please help me to resolve this problem?
Many thanks
javascript
add a comment |
I want to generate a .json file on my local project folder. I want to save the fetch API call response (which is an array of objects) into a .json file on my hard disc. Here is my code :
const fs = require('fs');
export default function getdirectory(token) {
return fetch(url, {
headers: {Authorization: "Bearer " + token}
})
.then((response) => response.json())
.catch((err) =>{
console.log("ERORRRRRRRR @@@@@@" + err);
})
.then((response) => {
//console.log(response);
var jsoncontent = JSON.stringify(response);
//console.log(jsoncontent);
fs.writeFileSync('files-to-cache.json', jsoncontent, function(err) {
if (err)
{console.log("EROROROROROR ****" + err);}
else
{ return response;}
});
// return response;
})
.catch((err) =>{
console.log("ERORRRRRRRR $$$$$$" + err);
})
};
When I run the code in vs code I get the following error:
undefined is not a function (near '...fs.writeFileSync...')
It seems that it happens because the data is not ready at the time of json file creation (asynchronous). Some web sites recommend setTimeOut function or sf.writeFileSync instead of sf.writeFile. I tried both, but still it doesn't work.
Would you please help me to resolve this problem?
Many thanks
javascript
I want to generate a .json file on my local project folder. I want to save the fetch API call response (which is an array of objects) into a .json file on my hard disc. Here is my code :
const fs = require('fs');
export default function getdirectory(token) {
return fetch(url, {
headers: {Authorization: "Bearer " + token}
})
.then((response) => response.json())
.catch((err) =>{
console.log("ERORRRRRRRR @@@@@@" + err);
})
.then((response) => {
//console.log(response);
var jsoncontent = JSON.stringify(response);
//console.log(jsoncontent);
fs.writeFileSync('files-to-cache.json', jsoncontent, function(err) {
if (err)
{console.log("EROROROROROR ****" + err);}
else
{ return response;}
});
// return response;
})
.catch((err) =>{
console.log("ERORRRRRRRR $$$$$$" + err);
})
};
When I run the code in vs code I get the following error:
undefined is not a function (near '...fs.writeFileSync...')
It seems that it happens because the data is not ready at the time of json file creation (asynchronous). Some web sites recommend setTimeOut function or sf.writeFileSync instead of sf.writeFile. I tried both, but still it doesn't work.
Would you please help me to resolve this problem?
Many thanks
javascript
javascript
asked Nov 15 '18 at 7:37


Glory GloryGlory Glory
1
1
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You are using the incorrect version of write file, there are two different types, see below:
The first is writeFileSync, this is synchronous, and will block the thread, meaning your script cannot continue until it is finished, but most importantly it doesn't accept a call back...
Try this instead:
fs.writeFile('data.json', jsonData, 'utf8', (err) => {
if (err) throw err
console.log('File created!`)
})
Hope this helps.
Thank you very much Lloyd for your guidance. I tried the code you provided, but unfortunately I still receive the same error : undefined is not a function (near '...fs.writeFile...')
– Glory Glory
Nov 15 '18 at 12:37
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53314472%2fhow-to-create-a-json-file-from-fetch-api-call-response-using-fs-writefile%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You are using the incorrect version of write file, there are two different types, see below:
The first is writeFileSync, this is synchronous, and will block the thread, meaning your script cannot continue until it is finished, but most importantly it doesn't accept a call back...
Try this instead:
fs.writeFile('data.json', jsonData, 'utf8', (err) => {
if (err) throw err
console.log('File created!`)
})
Hope this helps.
Thank you very much Lloyd for your guidance. I tried the code you provided, but unfortunately I still receive the same error : undefined is not a function (near '...fs.writeFile...')
– Glory Glory
Nov 15 '18 at 12:37
add a comment |
You are using the incorrect version of write file, there are two different types, see below:
The first is writeFileSync, this is synchronous, and will block the thread, meaning your script cannot continue until it is finished, but most importantly it doesn't accept a call back...
Try this instead:
fs.writeFile('data.json', jsonData, 'utf8', (err) => {
if (err) throw err
console.log('File created!`)
})
Hope this helps.
Thank you very much Lloyd for your guidance. I tried the code you provided, but unfortunately I still receive the same error : undefined is not a function (near '...fs.writeFile...')
– Glory Glory
Nov 15 '18 at 12:37
add a comment |
You are using the incorrect version of write file, there are two different types, see below:
The first is writeFileSync, this is synchronous, and will block the thread, meaning your script cannot continue until it is finished, but most importantly it doesn't accept a call back...
Try this instead:
fs.writeFile('data.json', jsonData, 'utf8', (err) => {
if (err) throw err
console.log('File created!`)
})
Hope this helps.
You are using the incorrect version of write file, there are two different types, see below:
The first is writeFileSync, this is synchronous, and will block the thread, meaning your script cannot continue until it is finished, but most importantly it doesn't accept a call back...
Try this instead:
fs.writeFile('data.json', jsonData, 'utf8', (err) => {
if (err) throw err
console.log('File created!`)
})
Hope this helps.
answered Nov 15 '18 at 8:15
LloydLloyd
916
916
Thank you very much Lloyd for your guidance. I tried the code you provided, but unfortunately I still receive the same error : undefined is not a function (near '...fs.writeFile...')
– Glory Glory
Nov 15 '18 at 12:37
add a comment |
Thank you very much Lloyd for your guidance. I tried the code you provided, but unfortunately I still receive the same error : undefined is not a function (near '...fs.writeFile...')
– Glory Glory
Nov 15 '18 at 12:37
Thank you very much Lloyd for your guidance. I tried the code you provided, but unfortunately I still receive the same error : undefined is not a function (near '...fs.writeFile...')
– Glory Glory
Nov 15 '18 at 12:37
Thank you very much Lloyd for your guidance. I tried the code you provided, but unfortunately I still receive the same error : undefined is not a function (near '...fs.writeFile...')
– Glory Glory
Nov 15 '18 at 12:37
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53314472%2fhow-to-create-a-json-file-from-fetch-api-call-response-using-fs-writefile%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
f2gG C2Xc nAz6FM0f,sBPQm,f9othZ9ceewjhZwVMVOprZwPshF,Fh