CakePHP group and count
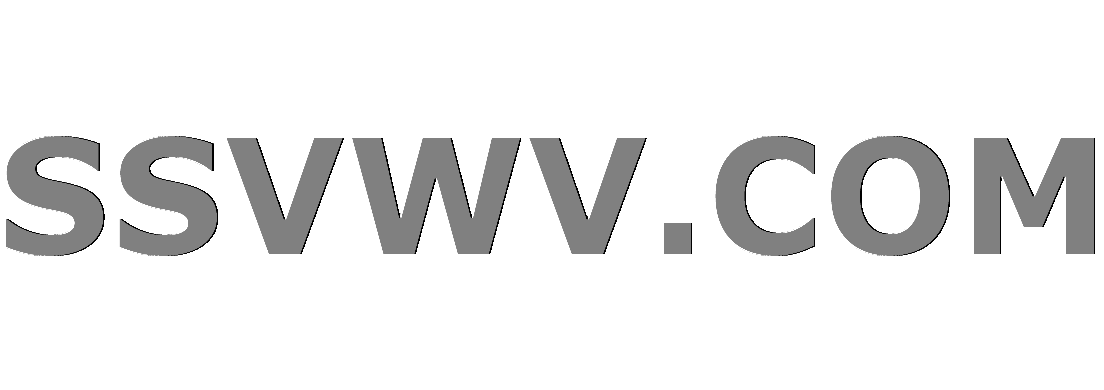
Multi tool use
I am having trouble figuring this out.
I have the following 3 tables: -
Transactions Table
- ID
- Date
- List item
Products Table
ID
Name
Price
Products_Transactions Table
Transaction_ID
Product_ID
Quantity
So the relationship is as follows - a transaction is made, and then the products_transactions table joins them together since a transaction can have multiple products and a product can have multiple transactions. The join_table also keeps track of the amount sold, so, for instance, a newspaper sells in transaction #1 and with a quantity of 2 (so 2 newspapers sold).
Now, I want to make a MySQL statement that finds all products sold, in a specific date interval, so I get something like this: -
- 3 x Newspapers
- 12 x Sodas
- 15 x Beer
So, it just counts and sums up all the products sold.
I have seriously tried everything - I am working with CakePHP so a solution provided in that would be helpful, but even just the plain SQL to achieve this might help me out.
So far, this is what I have: -
$productTransactionsTable = TableRegistry::get('products_transactions');
$productsTransactions = $productTransactionsTable->find('all');
$productsTransactions->matching('transactions', function ($q) {
return $q->where([
'transaction_date >=' => new DateTime('-1 week'),
'transaction_date <=' => new DateTime('now'),
'device_id IN' => $this->deviceIdsInDepartment(2)
]);
});
$productsTransactions->contain(['products']);
$productsTransactions->select([
'count' => $productsTransactions->func()->count('quantity'),
'name' => 'products.name'
]);
$productsTransactions->groupBy('products.id');
But this just gives out 1 single result that counts everything together into 1 row, like this:
/src/Controller/EconomyController.php (line 665)
[
(int) 0 => object(CakeORMEntity) {
'count' => (int) 4504,
'name' => 'D Morgenbrød',
'[new]' => false,
'[accessible]' => [
'*' => true
],
'[dirty]' => ,
'[original]' => ,
'[virtual]' => ,
'[errors]' => ,
'[invalid]' => ,
'[repository]' => 'products_transactions'
}
]
Any help is appreciated! I am seriously stuck here!
Thank you!
php mysql cakephp cakephp-3.x
add a comment |
I am having trouble figuring this out.
I have the following 3 tables: -
Transactions Table
- ID
- Date
- List item
Products Table
ID
Name
Price
Products_Transactions Table
Transaction_ID
Product_ID
Quantity
So the relationship is as follows - a transaction is made, and then the products_transactions table joins them together since a transaction can have multiple products and a product can have multiple transactions. The join_table also keeps track of the amount sold, so, for instance, a newspaper sells in transaction #1 and with a quantity of 2 (so 2 newspapers sold).
Now, I want to make a MySQL statement that finds all products sold, in a specific date interval, so I get something like this: -
- 3 x Newspapers
- 12 x Sodas
- 15 x Beer
So, it just counts and sums up all the products sold.
I have seriously tried everything - I am working with CakePHP so a solution provided in that would be helpful, but even just the plain SQL to achieve this might help me out.
So far, this is what I have: -
$productTransactionsTable = TableRegistry::get('products_transactions');
$productsTransactions = $productTransactionsTable->find('all');
$productsTransactions->matching('transactions', function ($q) {
return $q->where([
'transaction_date >=' => new DateTime('-1 week'),
'transaction_date <=' => new DateTime('now'),
'device_id IN' => $this->deviceIdsInDepartment(2)
]);
});
$productsTransactions->contain(['products']);
$productsTransactions->select([
'count' => $productsTransactions->func()->count('quantity'),
'name' => 'products.name'
]);
$productsTransactions->groupBy('products.id');
But this just gives out 1 single result that counts everything together into 1 row, like this:
/src/Controller/EconomyController.php (line 665)
[
(int) 0 => object(CakeORMEntity) {
'count' => (int) 4504,
'name' => 'D Morgenbrød',
'[new]' => false,
'[accessible]' => [
'*' => true
],
'[dirty]' => ,
'[original]' => ,
'[virtual]' => ,
'[errors]' => ,
'[invalid]' => ,
'[repository]' => 'products_transactions'
}
]
Any help is appreciated! I am seriously stuck here!
Thank you!
php mysql cakephp cakephp-3.x
add a comment |
I am having trouble figuring this out.
I have the following 3 tables: -
Transactions Table
- ID
- Date
- List item
Products Table
ID
Name
Price
Products_Transactions Table
Transaction_ID
Product_ID
Quantity
So the relationship is as follows - a transaction is made, and then the products_transactions table joins them together since a transaction can have multiple products and a product can have multiple transactions. The join_table also keeps track of the amount sold, so, for instance, a newspaper sells in transaction #1 and with a quantity of 2 (so 2 newspapers sold).
Now, I want to make a MySQL statement that finds all products sold, in a specific date interval, so I get something like this: -
- 3 x Newspapers
- 12 x Sodas
- 15 x Beer
So, it just counts and sums up all the products sold.
I have seriously tried everything - I am working with CakePHP so a solution provided in that would be helpful, but even just the plain SQL to achieve this might help me out.
So far, this is what I have: -
$productTransactionsTable = TableRegistry::get('products_transactions');
$productsTransactions = $productTransactionsTable->find('all');
$productsTransactions->matching('transactions', function ($q) {
return $q->where([
'transaction_date >=' => new DateTime('-1 week'),
'transaction_date <=' => new DateTime('now'),
'device_id IN' => $this->deviceIdsInDepartment(2)
]);
});
$productsTransactions->contain(['products']);
$productsTransactions->select([
'count' => $productsTransactions->func()->count('quantity'),
'name' => 'products.name'
]);
$productsTransactions->groupBy('products.id');
But this just gives out 1 single result that counts everything together into 1 row, like this:
/src/Controller/EconomyController.php (line 665)
[
(int) 0 => object(CakeORMEntity) {
'count' => (int) 4504,
'name' => 'D Morgenbrød',
'[new]' => false,
'[accessible]' => [
'*' => true
],
'[dirty]' => ,
'[original]' => ,
'[virtual]' => ,
'[errors]' => ,
'[invalid]' => ,
'[repository]' => 'products_transactions'
}
]
Any help is appreciated! I am seriously stuck here!
Thank you!
php mysql cakephp cakephp-3.x
I am having trouble figuring this out.
I have the following 3 tables: -
Transactions Table
- ID
- Date
- List item
Products Table
ID
Name
Price
Products_Transactions Table
Transaction_ID
Product_ID
Quantity
So the relationship is as follows - a transaction is made, and then the products_transactions table joins them together since a transaction can have multiple products and a product can have multiple transactions. The join_table also keeps track of the amount sold, so, for instance, a newspaper sells in transaction #1 and with a quantity of 2 (so 2 newspapers sold).
Now, I want to make a MySQL statement that finds all products sold, in a specific date interval, so I get something like this: -
- 3 x Newspapers
- 12 x Sodas
- 15 x Beer
So, it just counts and sums up all the products sold.
I have seriously tried everything - I am working with CakePHP so a solution provided in that would be helpful, but even just the plain SQL to achieve this might help me out.
So far, this is what I have: -
$productTransactionsTable = TableRegistry::get('products_transactions');
$productsTransactions = $productTransactionsTable->find('all');
$productsTransactions->matching('transactions', function ($q) {
return $q->where([
'transaction_date >=' => new DateTime('-1 week'),
'transaction_date <=' => new DateTime('now'),
'device_id IN' => $this->deviceIdsInDepartment(2)
]);
});
$productsTransactions->contain(['products']);
$productsTransactions->select([
'count' => $productsTransactions->func()->count('quantity'),
'name' => 'products.name'
]);
$productsTransactions->groupBy('products.id');
But this just gives out 1 single result that counts everything together into 1 row, like this:
/src/Controller/EconomyController.php (line 665)
[
(int) 0 => object(CakeORMEntity) {
'count' => (int) 4504,
'name' => 'D Morgenbrød',
'[new]' => false,
'[accessible]' => [
'*' => true
],
'[dirty]' => ,
'[original]' => ,
'[virtual]' => ,
'[errors]' => ,
'[invalid]' => ,
'[repository]' => 'products_transactions'
}
]
Any help is appreciated! I am seriously stuck here!
Thank you!
php mysql cakephp cakephp-3.x
php mysql cakephp cakephp-3.x
edited Nov 15 '18 at 11:58


Sayed Mohd Ali
1,4532519
1,4532519
asked Nov 15 '18 at 7:42
J.B.J.J.B.J.
185314
185314
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I think you are counting the total number of sold quantities and grouping so you are getting above results. I think, you need to try the following approach:
$data = $query
->select([
'name' => 'products.name',
'quantity' => 'products.quantity',
])
->group('products.id');
1
yea the problem was I that I used groupBy and not group -.-'
– J.B.J.
Nov 19 '18 at 5:48
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53314550%2fcakephp-group-and-count%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think you are counting the total number of sold quantities and grouping so you are getting above results. I think, you need to try the following approach:
$data = $query
->select([
'name' => 'products.name',
'quantity' => 'products.quantity',
])
->group('products.id');
1
yea the problem was I that I used groupBy and not group -.-'
– J.B.J.
Nov 19 '18 at 5:48
add a comment |
I think you are counting the total number of sold quantities and grouping so you are getting above results. I think, you need to try the following approach:
$data = $query
->select([
'name' => 'products.name',
'quantity' => 'products.quantity',
])
->group('products.id');
1
yea the problem was I that I used groupBy and not group -.-'
– J.B.J.
Nov 19 '18 at 5:48
add a comment |
I think you are counting the total number of sold quantities and grouping so you are getting above results. I think, you need to try the following approach:
$data = $query
->select([
'name' => 'products.name',
'quantity' => 'products.quantity',
])
->group('products.id');
I think you are counting the total number of sold quantities and grouping so you are getting above results. I think, you need to try the following approach:
$data = $query
->select([
'name' => 'products.name',
'quantity' => 'products.quantity',
])
->group('products.id');
answered Nov 15 '18 at 15:48
InvincibleInvincible
634614
634614
1
yea the problem was I that I used groupBy and not group -.-'
– J.B.J.
Nov 19 '18 at 5:48
add a comment |
1
yea the problem was I that I used groupBy and not group -.-'
– J.B.J.
Nov 19 '18 at 5:48
1
1
yea the problem was I that I used groupBy and not group -.-'
– J.B.J.
Nov 19 '18 at 5:48
yea the problem was I that I used groupBy and not group -.-'
– J.B.J.
Nov 19 '18 at 5:48
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53314550%2fcakephp-group-and-count%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
rRkcUq38ZbxjQBQ9Y26GQM,Ecu3h kHfH,cKLLj7Ff5 3fx,VQPCQ,yL hyYo q d 5Y1trdc2YdfeaG2Och 2,xZ 2TTo4 Z