How do I add data to a MongoDB database by clicking on a link or button without ngForm?
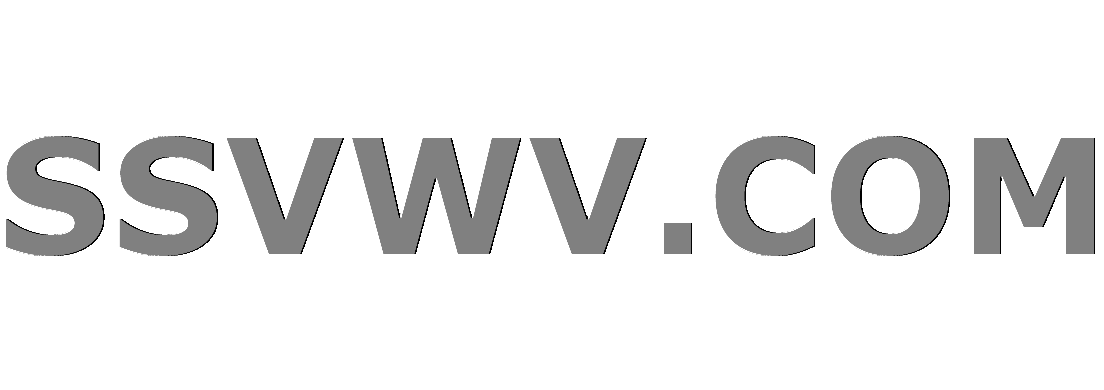
Multi tool use
up vote
0
down vote
favorite
I currently have this
<form #saveArticleForm="ngForm"(ngSubmit)="saveArticleForm.valid && saveArticle(saveArticleForm)">
<input type="text" #articles="ngModel" [(ngModel)]="userService.selectedUser.articles" name="articles" placeholder="Article"/>
<input type="submit" value="Save"/>
</form>
and this works fine. I'm able to save anything I type into an array in my database. However, I don't want the user to have to type the name of the article. They should be able to just click the save button and the title should be saved in the database.
I am able to get the name of the article using interpolation, {{article_title}}
, from a separate API that has our articles.
Extra information that may be useful
Front end
This is the saveArticle()
function
saveArticle(form: NgForm) {
this.userService.getUserProfile().subscribe(
res => {
this.userDetails = res["user"];
this.userService.addArticle(form.value).subscribe();
},
err => {}
);
}
These are addArticle()
and getUserProfile()
getUserProfile() {
return this.http.get(environment.apiBaseUrl + "/userProfile");
}
addArticle(title: string) {
return this.http.post(environment.apiBaseUrl + "/savearticle", title);
}
Back end
This is in the index.router.js
page
router.get("/userProfile", jwtHelper.verifyJwtToken, ctrlUser.userProfile);
router.post("/savearticle", ctrlUser.savearticle);
This is in the user.controller.js
page
module.exports.savearticle = (req, res, next) => {
User.findOneAndUpdate(
req._id,
{
$addToSet: { articles: req.body.articles }
},
{
new: true
},
function(err, savedArticle) {
if (err) {
res.send("Error saving article");
} else {
res.json(savedArticle);
}
}
);
};
module.exports.userProfile = (req, res, next) => {
User.findOne({ _id: req._id }, (err, user) => {
if (!user) {
return res
.status(404)
.json({ status: false, message: "User record not found." });
} else {
return res.status(200).json({
status: true,
user: _.pick(user, ["fullName", "email", "articles"])
});
}
});
};
html node.js angular typescript mean-stack
add a comment |
up vote
0
down vote
favorite
I currently have this
<form #saveArticleForm="ngForm"(ngSubmit)="saveArticleForm.valid && saveArticle(saveArticleForm)">
<input type="text" #articles="ngModel" [(ngModel)]="userService.selectedUser.articles" name="articles" placeholder="Article"/>
<input type="submit" value="Save"/>
</form>
and this works fine. I'm able to save anything I type into an array in my database. However, I don't want the user to have to type the name of the article. They should be able to just click the save button and the title should be saved in the database.
I am able to get the name of the article using interpolation, {{article_title}}
, from a separate API that has our articles.
Extra information that may be useful
Front end
This is the saveArticle()
function
saveArticle(form: NgForm) {
this.userService.getUserProfile().subscribe(
res => {
this.userDetails = res["user"];
this.userService.addArticle(form.value).subscribe();
},
err => {}
);
}
These are addArticle()
and getUserProfile()
getUserProfile() {
return this.http.get(environment.apiBaseUrl + "/userProfile");
}
addArticle(title: string) {
return this.http.post(environment.apiBaseUrl + "/savearticle", title);
}
Back end
This is in the index.router.js
page
router.get("/userProfile", jwtHelper.verifyJwtToken, ctrlUser.userProfile);
router.post("/savearticle", ctrlUser.savearticle);
This is in the user.controller.js
page
module.exports.savearticle = (req, res, next) => {
User.findOneAndUpdate(
req._id,
{
$addToSet: { articles: req.body.articles }
},
{
new: true
},
function(err, savedArticle) {
if (err) {
res.send("Error saving article");
} else {
res.json(savedArticle);
}
}
);
};
module.exports.userProfile = (req, res, next) => {
User.findOne({ _id: req._id }, (err, user) => {
if (!user) {
return res
.status(404)
.json({ status: false, message: "User record not found." });
} else {
return res.status(200).json({
status: true,
user: _.pick(user, ["fullName", "email", "articles"])
});
}
});
};
html node.js angular typescript mean-stack
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I currently have this
<form #saveArticleForm="ngForm"(ngSubmit)="saveArticleForm.valid && saveArticle(saveArticleForm)">
<input type="text" #articles="ngModel" [(ngModel)]="userService.selectedUser.articles" name="articles" placeholder="Article"/>
<input type="submit" value="Save"/>
</form>
and this works fine. I'm able to save anything I type into an array in my database. However, I don't want the user to have to type the name of the article. They should be able to just click the save button and the title should be saved in the database.
I am able to get the name of the article using interpolation, {{article_title}}
, from a separate API that has our articles.
Extra information that may be useful
Front end
This is the saveArticle()
function
saveArticle(form: NgForm) {
this.userService.getUserProfile().subscribe(
res => {
this.userDetails = res["user"];
this.userService.addArticle(form.value).subscribe();
},
err => {}
);
}
These are addArticle()
and getUserProfile()
getUserProfile() {
return this.http.get(environment.apiBaseUrl + "/userProfile");
}
addArticle(title: string) {
return this.http.post(environment.apiBaseUrl + "/savearticle", title);
}
Back end
This is in the index.router.js
page
router.get("/userProfile", jwtHelper.verifyJwtToken, ctrlUser.userProfile);
router.post("/savearticle", ctrlUser.savearticle);
This is in the user.controller.js
page
module.exports.savearticle = (req, res, next) => {
User.findOneAndUpdate(
req._id,
{
$addToSet: { articles: req.body.articles }
},
{
new: true
},
function(err, savedArticle) {
if (err) {
res.send("Error saving article");
} else {
res.json(savedArticle);
}
}
);
};
module.exports.userProfile = (req, res, next) => {
User.findOne({ _id: req._id }, (err, user) => {
if (!user) {
return res
.status(404)
.json({ status: false, message: "User record not found." });
} else {
return res.status(200).json({
status: true,
user: _.pick(user, ["fullName", "email", "articles"])
});
}
});
};
html node.js angular typescript mean-stack
I currently have this
<form #saveArticleForm="ngForm"(ngSubmit)="saveArticleForm.valid && saveArticle(saveArticleForm)">
<input type="text" #articles="ngModel" [(ngModel)]="userService.selectedUser.articles" name="articles" placeholder="Article"/>
<input type="submit" value="Save"/>
</form>
and this works fine. I'm able to save anything I type into an array in my database. However, I don't want the user to have to type the name of the article. They should be able to just click the save button and the title should be saved in the database.
I am able to get the name of the article using interpolation, {{article_title}}
, from a separate API that has our articles.
Extra information that may be useful
Front end
This is the saveArticle()
function
saveArticle(form: NgForm) {
this.userService.getUserProfile().subscribe(
res => {
this.userDetails = res["user"];
this.userService.addArticle(form.value).subscribe();
},
err => {}
);
}
These are addArticle()
and getUserProfile()
getUserProfile() {
return this.http.get(environment.apiBaseUrl + "/userProfile");
}
addArticle(title: string) {
return this.http.post(environment.apiBaseUrl + "/savearticle", title);
}
Back end
This is in the index.router.js
page
router.get("/userProfile", jwtHelper.verifyJwtToken, ctrlUser.userProfile);
router.post("/savearticle", ctrlUser.savearticle);
This is in the user.controller.js
page
module.exports.savearticle = (req, res, next) => {
User.findOneAndUpdate(
req._id,
{
$addToSet: { articles: req.body.articles }
},
{
new: true
},
function(err, savedArticle) {
if (err) {
res.send("Error saving article");
} else {
res.json(savedArticle);
}
}
);
};
module.exports.userProfile = (req, res, next) => {
User.findOne({ _id: req._id }, (err, user) => {
if (!user) {
return res
.status(404)
.json({ status: false, message: "User record not found." });
} else {
return res.status(200).json({
status: true,
user: _.pick(user, ["fullName", "email", "articles"])
});
}
});
};
html node.js angular typescript mean-stack
html node.js angular typescript mean-stack
asked Nov 11 at 5:03
Bryan Bastida
105
105
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245992%2fhow-do-i-add-data-to-a-mongodb-database-by-clicking-on-a-link-or-button-without%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
YiRtM2xgak4miAPibk9PC,jtSRnXL0vWbGlTlKfelKlvdVkfi