Custom ticks for seaborn heatmap
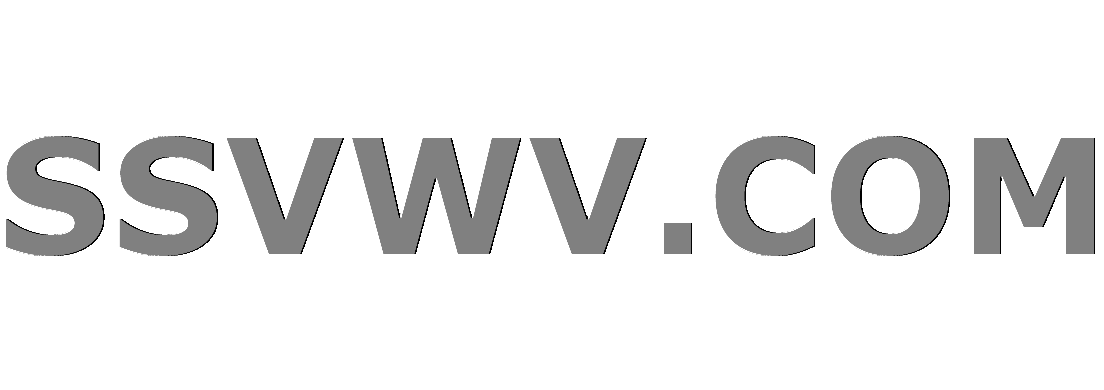
Multi tool use
up vote
0
down vote
favorite
I have some data that I would like to plot as a heatmap, it is essentially a 50x50 numpy array. As a result the heatmap axis labels range from 0 to 50, but actually I want the axis labels to go from -114 to 114 since this is the range of the data. When I set the tick labels however, they end up being bunched up on the axes (see image).
When I put in the lines
ax.set_xticks(ticks)
ax.set_yticks(ticks)
The heatmap ends up getting scaled (see image).
I have put in my code and some sample data, maybe someone can spot what I have done wrong.
import sys
import numpy as np
import pandas as pd
import matplotlib
import matplotlib.pyplot as plt
import os
import cv2 as cv
import seaborn as sns;
filepath = sys.argv[1]
drive, path_and_file = os.path.splitdrive(filepath)
path, file = os.path.split(path_and_file)
line_width = 3
font = {'family' : 'sans',
'weight' : 'normal',
'size' : 18}
matplotlib.rc('font', **font)
bagnames = ["hex_events_only.bag"]
groundtruth = [-92, 0]
noise_levels = ["-1.000000"]
rewards = ["sos"]
gt_angle = np.arctan2(groundtruth[0], groundtruth[1])
gt_mag = np.linalg.norm(groundtruth, axis=0)
print(gt_angle, gt_mag)
for bagname in bagnames:
print "==========", bagname, "=========="
for reward in rewards:
print " ---", reward, "--- "
for noise_level in noise_levels:
filename = filepath + "data_field_" + bagname + "_" + reward + "_" + noise_level
print filename
n_samples = (pd.read_csv(filename, delimiter="t", skiprows=1, names=["vx", "vy", "measure"])).values
x = n_samples[:, 0]
y = n_samples[:, 1]
z = n_samples[:, 2]
yrange = int(np.ptp(x))
xrange = int(np.ptp(y))
x_values = np.unique(x).size
y_values = np.unique(y).size
num_ticks = 10
ticks = np.linspace(int(-yrange/2.), int(yrange/2.), num_ticks, dtype=np.int)
img = np.reshape(z, (x_values, y_values))
img = img.T
img = cv.resize(img, (yrange, xrange))
savename = filepath + "hmap_" + bagname + "_" + reward + "_" + noise_level
fig, ax = plt.subplots()
img = cv.GaussianBlur(img, (5, 5), 0)
ax = sns.heatmap(img, cmap='viridis', yticklabels=ticks, xticklabels=ticks)
# ax.set_xticks(ticks)
# ax.set_yticks(ticks)
# ax.axvline(groundtruth[0], linestyle='--', c='r', linewidth=line_width)
# ax.axhline(groundtruth[1], linestyle='--', c='r', linewidth=line_width)
plt.show()
fig.savefig(savename + ".png", transparent=True, bbox_inches='tight', pad_inches=0)
plt.close()
https://1drv.ms/u/s!Ap0up1KFhZOughZ3dx9rwq-9yiF9
python matplotlib seaborn heatmap
add a comment |
up vote
0
down vote
favorite
I have some data that I would like to plot as a heatmap, it is essentially a 50x50 numpy array. As a result the heatmap axis labels range from 0 to 50, but actually I want the axis labels to go from -114 to 114 since this is the range of the data. When I set the tick labels however, they end up being bunched up on the axes (see image).
When I put in the lines
ax.set_xticks(ticks)
ax.set_yticks(ticks)
The heatmap ends up getting scaled (see image).
I have put in my code and some sample data, maybe someone can spot what I have done wrong.
import sys
import numpy as np
import pandas as pd
import matplotlib
import matplotlib.pyplot as plt
import os
import cv2 as cv
import seaborn as sns;
filepath = sys.argv[1]
drive, path_and_file = os.path.splitdrive(filepath)
path, file = os.path.split(path_and_file)
line_width = 3
font = {'family' : 'sans',
'weight' : 'normal',
'size' : 18}
matplotlib.rc('font', **font)
bagnames = ["hex_events_only.bag"]
groundtruth = [-92, 0]
noise_levels = ["-1.000000"]
rewards = ["sos"]
gt_angle = np.arctan2(groundtruth[0], groundtruth[1])
gt_mag = np.linalg.norm(groundtruth, axis=0)
print(gt_angle, gt_mag)
for bagname in bagnames:
print "==========", bagname, "=========="
for reward in rewards:
print " ---", reward, "--- "
for noise_level in noise_levels:
filename = filepath + "data_field_" + bagname + "_" + reward + "_" + noise_level
print filename
n_samples = (pd.read_csv(filename, delimiter="t", skiprows=1, names=["vx", "vy", "measure"])).values
x = n_samples[:, 0]
y = n_samples[:, 1]
z = n_samples[:, 2]
yrange = int(np.ptp(x))
xrange = int(np.ptp(y))
x_values = np.unique(x).size
y_values = np.unique(y).size
num_ticks = 10
ticks = np.linspace(int(-yrange/2.), int(yrange/2.), num_ticks, dtype=np.int)
img = np.reshape(z, (x_values, y_values))
img = img.T
img = cv.resize(img, (yrange, xrange))
savename = filepath + "hmap_" + bagname + "_" + reward + "_" + noise_level
fig, ax = plt.subplots()
img = cv.GaussianBlur(img, (5, 5), 0)
ax = sns.heatmap(img, cmap='viridis', yticklabels=ticks, xticklabels=ticks)
# ax.set_xticks(ticks)
# ax.set_yticks(ticks)
# ax.axvline(groundtruth[0], linestyle='--', c='r', linewidth=line_width)
# ax.axhline(groundtruth[1], linestyle='--', c='r', linewidth=line_width)
plt.show()
fig.savefig(savename + ".png", transparent=True, bbox_inches='tight', pad_inches=0)
plt.close()
https://1drv.ms/u/s!Ap0up1KFhZOughZ3dx9rwq-9yiF9
python matplotlib seaborn heatmap
1
You do not want to use asns.heatmap
here. Depending on whether the grid is defined on the centers or edges you want aplt.imshow
orplt.pcolormesh
plot.
– ImportanceOfBeingErnest
Nov 11 at 12:13
@ImportanceOfBeingErnest Why exactly?
– Mr Squid
Nov 11 at 13:22
1
Because it's simply not meant to be used for this purpose.searborn.heatmap
uses apcolormesh
internally and manipulates it in a way to be useful to show categorical plots. Here you do not have a categorical plot. Hence it's much easier to directly use apcolormesh
plot (orimshow
actually, depending on the desired grid), instead of externally trying to revert all the changesheatmap
makes to this internally.
– ImportanceOfBeingErnest
Nov 11 at 13:25
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have some data that I would like to plot as a heatmap, it is essentially a 50x50 numpy array. As a result the heatmap axis labels range from 0 to 50, but actually I want the axis labels to go from -114 to 114 since this is the range of the data. When I set the tick labels however, they end up being bunched up on the axes (see image).
When I put in the lines
ax.set_xticks(ticks)
ax.set_yticks(ticks)
The heatmap ends up getting scaled (see image).
I have put in my code and some sample data, maybe someone can spot what I have done wrong.
import sys
import numpy as np
import pandas as pd
import matplotlib
import matplotlib.pyplot as plt
import os
import cv2 as cv
import seaborn as sns;
filepath = sys.argv[1]
drive, path_and_file = os.path.splitdrive(filepath)
path, file = os.path.split(path_and_file)
line_width = 3
font = {'family' : 'sans',
'weight' : 'normal',
'size' : 18}
matplotlib.rc('font', **font)
bagnames = ["hex_events_only.bag"]
groundtruth = [-92, 0]
noise_levels = ["-1.000000"]
rewards = ["sos"]
gt_angle = np.arctan2(groundtruth[0], groundtruth[1])
gt_mag = np.linalg.norm(groundtruth, axis=0)
print(gt_angle, gt_mag)
for bagname in bagnames:
print "==========", bagname, "=========="
for reward in rewards:
print " ---", reward, "--- "
for noise_level in noise_levels:
filename = filepath + "data_field_" + bagname + "_" + reward + "_" + noise_level
print filename
n_samples = (pd.read_csv(filename, delimiter="t", skiprows=1, names=["vx", "vy", "measure"])).values
x = n_samples[:, 0]
y = n_samples[:, 1]
z = n_samples[:, 2]
yrange = int(np.ptp(x))
xrange = int(np.ptp(y))
x_values = np.unique(x).size
y_values = np.unique(y).size
num_ticks = 10
ticks = np.linspace(int(-yrange/2.), int(yrange/2.), num_ticks, dtype=np.int)
img = np.reshape(z, (x_values, y_values))
img = img.T
img = cv.resize(img, (yrange, xrange))
savename = filepath + "hmap_" + bagname + "_" + reward + "_" + noise_level
fig, ax = plt.subplots()
img = cv.GaussianBlur(img, (5, 5), 0)
ax = sns.heatmap(img, cmap='viridis', yticklabels=ticks, xticklabels=ticks)
# ax.set_xticks(ticks)
# ax.set_yticks(ticks)
# ax.axvline(groundtruth[0], linestyle='--', c='r', linewidth=line_width)
# ax.axhline(groundtruth[1], linestyle='--', c='r', linewidth=line_width)
plt.show()
fig.savefig(savename + ".png", transparent=True, bbox_inches='tight', pad_inches=0)
plt.close()
https://1drv.ms/u/s!Ap0up1KFhZOughZ3dx9rwq-9yiF9
python matplotlib seaborn heatmap
I have some data that I would like to plot as a heatmap, it is essentially a 50x50 numpy array. As a result the heatmap axis labels range from 0 to 50, but actually I want the axis labels to go from -114 to 114 since this is the range of the data. When I set the tick labels however, they end up being bunched up on the axes (see image).
When I put in the lines
ax.set_xticks(ticks)
ax.set_yticks(ticks)
The heatmap ends up getting scaled (see image).
I have put in my code and some sample data, maybe someone can spot what I have done wrong.
import sys
import numpy as np
import pandas as pd
import matplotlib
import matplotlib.pyplot as plt
import os
import cv2 as cv
import seaborn as sns;
filepath = sys.argv[1]
drive, path_and_file = os.path.splitdrive(filepath)
path, file = os.path.split(path_and_file)
line_width = 3
font = {'family' : 'sans',
'weight' : 'normal',
'size' : 18}
matplotlib.rc('font', **font)
bagnames = ["hex_events_only.bag"]
groundtruth = [-92, 0]
noise_levels = ["-1.000000"]
rewards = ["sos"]
gt_angle = np.arctan2(groundtruth[0], groundtruth[1])
gt_mag = np.linalg.norm(groundtruth, axis=0)
print(gt_angle, gt_mag)
for bagname in bagnames:
print "==========", bagname, "=========="
for reward in rewards:
print " ---", reward, "--- "
for noise_level in noise_levels:
filename = filepath + "data_field_" + bagname + "_" + reward + "_" + noise_level
print filename
n_samples = (pd.read_csv(filename, delimiter="t", skiprows=1, names=["vx", "vy", "measure"])).values
x = n_samples[:, 0]
y = n_samples[:, 1]
z = n_samples[:, 2]
yrange = int(np.ptp(x))
xrange = int(np.ptp(y))
x_values = np.unique(x).size
y_values = np.unique(y).size
num_ticks = 10
ticks = np.linspace(int(-yrange/2.), int(yrange/2.), num_ticks, dtype=np.int)
img = np.reshape(z, (x_values, y_values))
img = img.T
img = cv.resize(img, (yrange, xrange))
savename = filepath + "hmap_" + bagname + "_" + reward + "_" + noise_level
fig, ax = plt.subplots()
img = cv.GaussianBlur(img, (5, 5), 0)
ax = sns.heatmap(img, cmap='viridis', yticklabels=ticks, xticklabels=ticks)
# ax.set_xticks(ticks)
# ax.set_yticks(ticks)
# ax.axvline(groundtruth[0], linestyle='--', c='r', linewidth=line_width)
# ax.axhline(groundtruth[1], linestyle='--', c='r', linewidth=line_width)
plt.show()
fig.savefig(savename + ".png", transparent=True, bbox_inches='tight', pad_inches=0)
plt.close()
https://1drv.ms/u/s!Ap0up1KFhZOughZ3dx9rwq-9yiF9
python matplotlib seaborn heatmap
python matplotlib seaborn heatmap
asked Nov 11 at 11:18
Mr Squid
10918
10918
1
You do not want to use asns.heatmap
here. Depending on whether the grid is defined on the centers or edges you want aplt.imshow
orplt.pcolormesh
plot.
– ImportanceOfBeingErnest
Nov 11 at 12:13
@ImportanceOfBeingErnest Why exactly?
– Mr Squid
Nov 11 at 13:22
1
Because it's simply not meant to be used for this purpose.searborn.heatmap
uses apcolormesh
internally and manipulates it in a way to be useful to show categorical plots. Here you do not have a categorical plot. Hence it's much easier to directly use apcolormesh
plot (orimshow
actually, depending on the desired grid), instead of externally trying to revert all the changesheatmap
makes to this internally.
– ImportanceOfBeingErnest
Nov 11 at 13:25
add a comment |
1
You do not want to use asns.heatmap
here. Depending on whether the grid is defined on the centers or edges you want aplt.imshow
orplt.pcolormesh
plot.
– ImportanceOfBeingErnest
Nov 11 at 12:13
@ImportanceOfBeingErnest Why exactly?
– Mr Squid
Nov 11 at 13:22
1
Because it's simply not meant to be used for this purpose.searborn.heatmap
uses apcolormesh
internally and manipulates it in a way to be useful to show categorical plots. Here you do not have a categorical plot. Hence it's much easier to directly use apcolormesh
plot (orimshow
actually, depending on the desired grid), instead of externally trying to revert all the changesheatmap
makes to this internally.
– ImportanceOfBeingErnest
Nov 11 at 13:25
1
1
You do not want to use a
sns.heatmap
here. Depending on whether the grid is defined on the centers or edges you want a plt.imshow
or plt.pcolormesh
plot.– ImportanceOfBeingErnest
Nov 11 at 12:13
You do not want to use a
sns.heatmap
here. Depending on whether the grid is defined on the centers or edges you want a plt.imshow
or plt.pcolormesh
plot.– ImportanceOfBeingErnest
Nov 11 at 12:13
@ImportanceOfBeingErnest Why exactly?
– Mr Squid
Nov 11 at 13:22
@ImportanceOfBeingErnest Why exactly?
– Mr Squid
Nov 11 at 13:22
1
1
Because it's simply not meant to be used for this purpose.
searborn.heatmap
uses a pcolormesh
internally and manipulates it in a way to be useful to show categorical plots. Here you do not have a categorical plot. Hence it's much easier to directly use a pcolormesh
plot (or imshow
actually, depending on the desired grid), instead of externally trying to revert all the changes heatmap
makes to this internally.– ImportanceOfBeingErnest
Nov 11 at 13:25
Because it's simply not meant to be used for this purpose.
searborn.heatmap
uses a pcolormesh
internally and manipulates it in a way to be useful to show categorical plots. Here you do not have a categorical plot. Hence it's much easier to directly use a pcolormesh
plot (or imshow
actually, depending on the desired grid), instead of externally trying to revert all the changes heatmap
makes to this internally.– ImportanceOfBeingErnest
Nov 11 at 13:25
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
@ImportanceOfBeingErnest pointed out to me that the approach of using Seaborn was wrong in the first place (see comments). So I changed the approach, which now works exactly as I want it to. In case anyone else runs into this problem, the following code with generate a heatmap from data:
import numpy as np
import pandas as pd
import matplotlib
import matplotlib.pyplot as plt
import cv2 as cv
font = {'family' : 'sans',
'weight' : 'normal',
'size' : 18}
matplotlib.rc('font', **font)
filepath = "path/to/data/"
dataname = "data.txt"
filename = filepath + dataname
n_samples = (pd.read_csv(filename, delimiter="t", skiprows=1, names=["x", "y", "value"])).values
x = n_samples[:, 0]
y = n_samples[:, 1]
z = n_samples[:, 2]
line_width = 2
yrange = int(np.ptp(x))
xrange = int(np.ptp(y))
x_values = np.unique(x).size
y_values = np.unique(y).size
num_ticks = 10
ticks = np.linspace(int(-yrange/2.), int(yrange/2.), num_ticks, dtype=np.int)
img = np.reshape(z, (x_values, y_values))
img = img.T
img = cv.resize(img, (yrange, xrange))
fig, ax = plt.subplots()
im = ax.imshow(img, cmap='viridis', extent=[-xrange/2., xrange/2., -yrange/2., yrange/2.])
ax.axvline(groundtruth[0], linestyle='--', c='r', linewidth=line_width)
ax.axhline(groundtruth[1], linestyle='--', c='r', linewidth=line_width)
ax.set_xlabel("$v_x$")
ax.set_ylabel("$v_y$")
cbar = fig.colorbar(im)
cbar.ax.set_yticklabels([''])
cbar.ax.set_ylabel('Reward')
fig.tight_layout()
savename = filepath + "hmap_" + bagname + "_" + reward + "_" + noise_level
fig.savefig(savename + ".pdf", transparent=True, bbox_inches='tight', pad_inches=0)
plt.close()
# plt.show()
Here's what the output is like:
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
@ImportanceOfBeingErnest pointed out to me that the approach of using Seaborn was wrong in the first place (see comments). So I changed the approach, which now works exactly as I want it to. In case anyone else runs into this problem, the following code with generate a heatmap from data:
import numpy as np
import pandas as pd
import matplotlib
import matplotlib.pyplot as plt
import cv2 as cv
font = {'family' : 'sans',
'weight' : 'normal',
'size' : 18}
matplotlib.rc('font', **font)
filepath = "path/to/data/"
dataname = "data.txt"
filename = filepath + dataname
n_samples = (pd.read_csv(filename, delimiter="t", skiprows=1, names=["x", "y", "value"])).values
x = n_samples[:, 0]
y = n_samples[:, 1]
z = n_samples[:, 2]
line_width = 2
yrange = int(np.ptp(x))
xrange = int(np.ptp(y))
x_values = np.unique(x).size
y_values = np.unique(y).size
num_ticks = 10
ticks = np.linspace(int(-yrange/2.), int(yrange/2.), num_ticks, dtype=np.int)
img = np.reshape(z, (x_values, y_values))
img = img.T
img = cv.resize(img, (yrange, xrange))
fig, ax = plt.subplots()
im = ax.imshow(img, cmap='viridis', extent=[-xrange/2., xrange/2., -yrange/2., yrange/2.])
ax.axvline(groundtruth[0], linestyle='--', c='r', linewidth=line_width)
ax.axhline(groundtruth[1], linestyle='--', c='r', linewidth=line_width)
ax.set_xlabel("$v_x$")
ax.set_ylabel("$v_y$")
cbar = fig.colorbar(im)
cbar.ax.set_yticklabels([''])
cbar.ax.set_ylabel('Reward')
fig.tight_layout()
savename = filepath + "hmap_" + bagname + "_" + reward + "_" + noise_level
fig.savefig(savename + ".pdf", transparent=True, bbox_inches='tight', pad_inches=0)
plt.close()
# plt.show()
Here's what the output is like:
add a comment |
up vote
0
down vote
accepted
@ImportanceOfBeingErnest pointed out to me that the approach of using Seaborn was wrong in the first place (see comments). So I changed the approach, which now works exactly as I want it to. In case anyone else runs into this problem, the following code with generate a heatmap from data:
import numpy as np
import pandas as pd
import matplotlib
import matplotlib.pyplot as plt
import cv2 as cv
font = {'family' : 'sans',
'weight' : 'normal',
'size' : 18}
matplotlib.rc('font', **font)
filepath = "path/to/data/"
dataname = "data.txt"
filename = filepath + dataname
n_samples = (pd.read_csv(filename, delimiter="t", skiprows=1, names=["x", "y", "value"])).values
x = n_samples[:, 0]
y = n_samples[:, 1]
z = n_samples[:, 2]
line_width = 2
yrange = int(np.ptp(x))
xrange = int(np.ptp(y))
x_values = np.unique(x).size
y_values = np.unique(y).size
num_ticks = 10
ticks = np.linspace(int(-yrange/2.), int(yrange/2.), num_ticks, dtype=np.int)
img = np.reshape(z, (x_values, y_values))
img = img.T
img = cv.resize(img, (yrange, xrange))
fig, ax = plt.subplots()
im = ax.imshow(img, cmap='viridis', extent=[-xrange/2., xrange/2., -yrange/2., yrange/2.])
ax.axvline(groundtruth[0], linestyle='--', c='r', linewidth=line_width)
ax.axhline(groundtruth[1], linestyle='--', c='r', linewidth=line_width)
ax.set_xlabel("$v_x$")
ax.set_ylabel("$v_y$")
cbar = fig.colorbar(im)
cbar.ax.set_yticklabels([''])
cbar.ax.set_ylabel('Reward')
fig.tight_layout()
savename = filepath + "hmap_" + bagname + "_" + reward + "_" + noise_level
fig.savefig(savename + ".pdf", transparent=True, bbox_inches='tight', pad_inches=0)
plt.close()
# plt.show()
Here's what the output is like:
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
@ImportanceOfBeingErnest pointed out to me that the approach of using Seaborn was wrong in the first place (see comments). So I changed the approach, which now works exactly as I want it to. In case anyone else runs into this problem, the following code with generate a heatmap from data:
import numpy as np
import pandas as pd
import matplotlib
import matplotlib.pyplot as plt
import cv2 as cv
font = {'family' : 'sans',
'weight' : 'normal',
'size' : 18}
matplotlib.rc('font', **font)
filepath = "path/to/data/"
dataname = "data.txt"
filename = filepath + dataname
n_samples = (pd.read_csv(filename, delimiter="t", skiprows=1, names=["x", "y", "value"])).values
x = n_samples[:, 0]
y = n_samples[:, 1]
z = n_samples[:, 2]
line_width = 2
yrange = int(np.ptp(x))
xrange = int(np.ptp(y))
x_values = np.unique(x).size
y_values = np.unique(y).size
num_ticks = 10
ticks = np.linspace(int(-yrange/2.), int(yrange/2.), num_ticks, dtype=np.int)
img = np.reshape(z, (x_values, y_values))
img = img.T
img = cv.resize(img, (yrange, xrange))
fig, ax = plt.subplots()
im = ax.imshow(img, cmap='viridis', extent=[-xrange/2., xrange/2., -yrange/2., yrange/2.])
ax.axvline(groundtruth[0], linestyle='--', c='r', linewidth=line_width)
ax.axhline(groundtruth[1], linestyle='--', c='r', linewidth=line_width)
ax.set_xlabel("$v_x$")
ax.set_ylabel("$v_y$")
cbar = fig.colorbar(im)
cbar.ax.set_yticklabels([''])
cbar.ax.set_ylabel('Reward')
fig.tight_layout()
savename = filepath + "hmap_" + bagname + "_" + reward + "_" + noise_level
fig.savefig(savename + ".pdf", transparent=True, bbox_inches='tight', pad_inches=0)
plt.close()
# plt.show()
Here's what the output is like:
@ImportanceOfBeingErnest pointed out to me that the approach of using Seaborn was wrong in the first place (see comments). So I changed the approach, which now works exactly as I want it to. In case anyone else runs into this problem, the following code with generate a heatmap from data:
import numpy as np
import pandas as pd
import matplotlib
import matplotlib.pyplot as plt
import cv2 as cv
font = {'family' : 'sans',
'weight' : 'normal',
'size' : 18}
matplotlib.rc('font', **font)
filepath = "path/to/data/"
dataname = "data.txt"
filename = filepath + dataname
n_samples = (pd.read_csv(filename, delimiter="t", skiprows=1, names=["x", "y", "value"])).values
x = n_samples[:, 0]
y = n_samples[:, 1]
z = n_samples[:, 2]
line_width = 2
yrange = int(np.ptp(x))
xrange = int(np.ptp(y))
x_values = np.unique(x).size
y_values = np.unique(y).size
num_ticks = 10
ticks = np.linspace(int(-yrange/2.), int(yrange/2.), num_ticks, dtype=np.int)
img = np.reshape(z, (x_values, y_values))
img = img.T
img = cv.resize(img, (yrange, xrange))
fig, ax = plt.subplots()
im = ax.imshow(img, cmap='viridis', extent=[-xrange/2., xrange/2., -yrange/2., yrange/2.])
ax.axvline(groundtruth[0], linestyle='--', c='r', linewidth=line_width)
ax.axhline(groundtruth[1], linestyle='--', c='r', linewidth=line_width)
ax.set_xlabel("$v_x$")
ax.set_ylabel("$v_y$")
cbar = fig.colorbar(im)
cbar.ax.set_yticklabels([''])
cbar.ax.set_ylabel('Reward')
fig.tight_layout()
savename = filepath + "hmap_" + bagname + "_" + reward + "_" + noise_level
fig.savefig(savename + ".pdf", transparent=True, bbox_inches='tight', pad_inches=0)
plt.close()
# plt.show()
Here's what the output is like:
answered Nov 11 at 14:19
Mr Squid
10918
10918
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53248186%2fcustom-ticks-for-seaborn-heatmap%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
z0cnaO DBskB,kPqbTx64JV,uG3WkoNc VrKBuTSuLnfir 24iAeFopq aTIS
1
You do not want to use a
sns.heatmap
here. Depending on whether the grid is defined on the centers or edges you want aplt.imshow
orplt.pcolormesh
plot.– ImportanceOfBeingErnest
Nov 11 at 12:13
@ImportanceOfBeingErnest Why exactly?
– Mr Squid
Nov 11 at 13:22
1
Because it's simply not meant to be used for this purpose.
searborn.heatmap
uses apcolormesh
internally and manipulates it in a way to be useful to show categorical plots. Here you do not have a categorical plot. Hence it's much easier to directly use apcolormesh
plot (orimshow
actually, depending on the desired grid), instead of externally trying to revert all the changesheatmap
makes to this internally.– ImportanceOfBeingErnest
Nov 11 at 13:25